Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial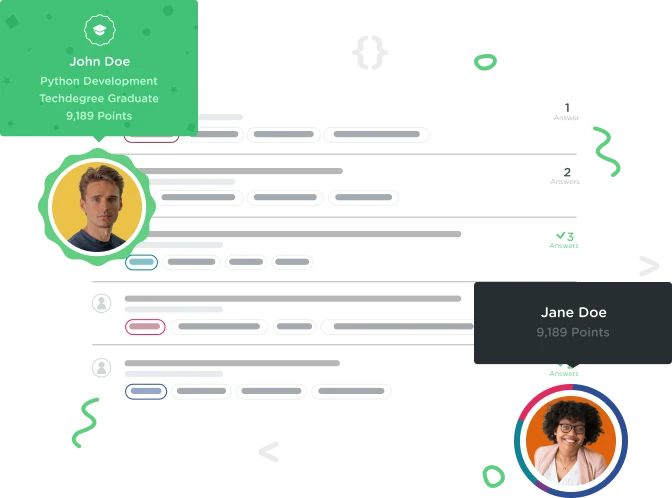

Chastin Davis
Courses Plus Student 2,299 PointsWho do I put two iterables into a tuple and then a list of tuples?
I seem to have trouble getting the tuple formed to even get to the list stage.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(list_one, list_two):
tupled= ()
indexed = 0
for index, item1 in enumerate(list_one, start = 0):
for index, item2 in enumerate(list_two, start = 0):
tupled = (("{}, {}").format(item1, item2))
print (tupled)
return tupled
Damon Edstrom
Courses Plus Student 269 PointsDamon Edstrom
Courses Plus Student 269 PointsYou shouldn't need to do two enumerates since in the instructions it specifies that you should assume the inputs will be the same length. To add when you do this you will get back twice the amount of results that you want, the first iteration over enumerate will return a single value from list_one while returning all sequentially form list two.
The specific reason you aren't getting a tuple I believe is because in your format you are only putting a single string in parenthesis, you need the brackets in a separate quote pair and the comma shouldn't be in quotes.
You also don't need to store the tuple outside of the inner function since you're just appending it to the list and dumping it. You're already creating 'tupled' inside of the inner function as well so it servers a null purpose. Not sure what indexed is doing there either. Another thing to note is that python by default starts a 0 so you shouldn't need to specify the starting point in your enumerate functions.
Working solution if you wanted to use it as reference and see how you can alter your own code to do the challenge: