Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial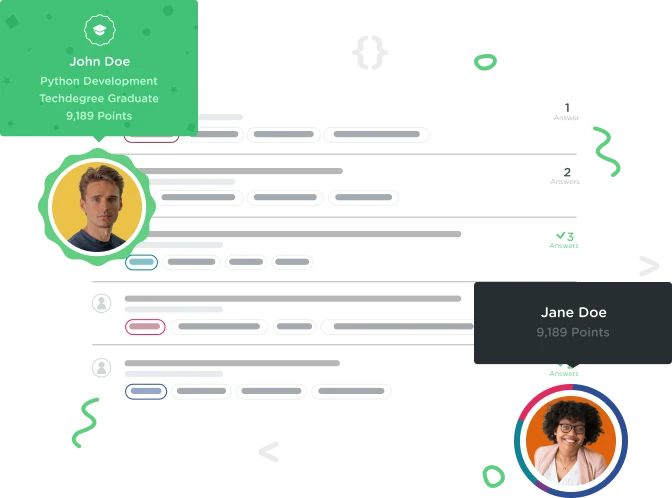
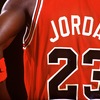
Serdar Halac
15,259 PointsWhta is the point of the /static/ directory?
Why is there a STATIC_URL setting in settings.py? How is it different from STATICFILES_DIRS?
Here:
STATICFILES_DIRS = (
os.path.join(BASE_DIR, 'assets'),
)
We are basically saying "ok, so now Django Basics/learning_site/assets/ is where the static files are! Just so you know", but then why is there a STATIC_URL setting that seems to create a directory where our static folders are?
STATIC_URL = '/static/'
What is the code above for? Why are we making two directories for static files?
Also, what is debug mode?
And when our code had this in urls.py:
urlpatterns = [
url(r'^courses/', include('courses.urls')),
url(r'^admin/', include(admin.site.urls)),
url(r'^$', views.hello_world),
]
urlpatterns += staticfiles_urlpatterns()
Is that adding the directory /static/ to the list of URLS? Does the staticfiles_urlpatterns() function look in settings.py for whatever the STATIC_URL setting is set to?
Also, in the HTML folder, what is the point of the static tag?
<link rel="stylesheet" href="{% static 'css/layout.css' %}">
What does the static tag do? href= css/layout.css should work just fine, no? Why is the static tag there? What is it doing that no static tag would not be able to do? I thought rel="stylesheet" was how the HTML file knew that the href pointed to a css file
I know it's a lot of questions, but I've just need a bunch of clearing up!
[MOD: added code formatting -cf]
3 Answers
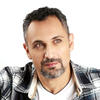
Haydar Al-Rikabi
5,971 PointsThere are two steps to it:
The template tag {% static 'path_to_static_file_within_staticfiles_dir' %} tells Django's template engine to prefix the path within this tag with STATIC_URL. So, the rendered template will have the following format: STATIC_URL/path_to_static_file_within_staticfiles_dir For example: /static/css/layout.css
Then when you deploy the project on a server, Django looks into the href and src parameters of these html tags:
- css <link rel="stylesheet" type="text/css" href="url_to_file">
- javascript <script type="text/javascript" src="url_to_file">
- image <img src="url_to_file">
and replaces the value of STATIC_URL (in this case it is '/static/') with either of two urt paths:
- On a development server, /static/ will be replaced by the related path in the default static directory of the project and the related app (STATIC_URL is also considered the default folder). If it couldn't find the default directory, then Django will look for the static files in STATICFILES_DIRS at runtime, i.e. it will not be printed in the template.
- On a production server, /static/ will be replaced by a url path that conforms to the rules of the running server. This also happens at runtime. So, if you inspect the web page source code, you will find /static/ is still showing there.
Please note that STATIC_URL is required by Django. You cannot leave it empty and it must always be in the following format: STATIC_URL = '/any_string_here/'
One more thing. You don't need to add the following lines to the project's url.py in Django 2.0 because Django knows that it has to prefix the static file url path in the template with STATIC_URL:
from django.contrib.staticfiles.urls import staticfiles_urlpatterns
urlpatterns += staticfiles_urlpatterns()
As for this line at the top of a template: {% load static from staticfiles %}
The {% load %} tag tells Django template engine to look for the "static" method inside your INSTALLED_APPS in settings.py.
In the Django’s repository on Github, the “static” method lives here: django/contrib/staticfiles/templatetags/staticfiles.py
- django/contrib/staticfiles is the installed app in settings.py
- /templatetags because "static" is loaded in a template
- /staticfiles.py is the name of the file where the loaded "static" method lives.
Prior to Django 2.0 we had to explicitly tell the template engine in which file "static" lives. Hence:
{% load static from staticfiles %} # load the static method from staticfiles.py
But Django 2.0 requires only the name of the method (template tag) and it will find it for us inside the templatetags folder of the installed app (for example: django.contrib.staticfiles in settings.py). So, now this is all what you need to make it work:
{% load static %} # Django knows where to find the static method / template tag
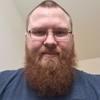
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsNo idea about the STATIC_URL but have a look at the docs: https://docs.djangoproject.com/en/2.0/ref/settings/#std:setting-STATIC_URL
When DEBUG is set to True you get a lot of nice information when your app crashes to help you figure out what the problem might be - if set to False (=production mode) you wont get those details, all you get might be a white page with a <h1> saying Bad Request (500)
I think it might be a good idea for you to have a look at the docs: https://docs.djangoproject.com/en/2.0/ (just change 2.0 to what ever version you are using)
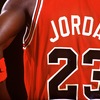
Serdar Halac
15,259 PointsYeah, I've been checking out the docs. Learning how to go through this stuff is a big part of being a developer I suppose.
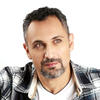
Haydar Al-Rikabi
5,971 PointsPlease check my updated answer for further explanation.
Can Chris Freeman check out my answer and comment on it.
Serdar Halac
15,259 PointsSerdar Halac
15,259 PointsGreat answer. Thank you very much for the detailed explanation.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 Pointsgreat answer!