Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial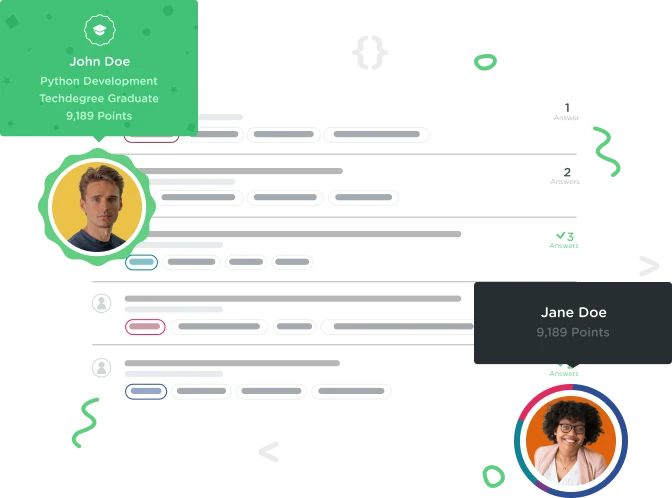
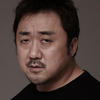
chanjong kim
2,315 Pointswhy?
class Department
{
public int Id { get; set; }
public string Name { get; set; }
}
Department dept1 = new Department() { Id = 1004, Name = "R&D" };
Department dept2 = new Department() { Id = 1004, Name = "R&D" };
dept1.Equals(dept2);
why answer is false?? Equals is not overriden, so it evaluates if dept1 is same object as dept2. both dept1 and dept2 are Department object, so I thought it returns true, But answer is false, why?
1 Answer

andren
28,558 Pointsdept1
and dept2
are both objects created from the department
class, but they are not the same object. The Equal
method checks if two objects are the same instance of a class, not that they were built from the same class.
When the new
keyword is used you create a new object from a class, which has its own state and own place in memory where it is stored. That's why you can change their properties independently of each other. In other words changing the Id
of dept1
won't change the Id
of dept2
and so on.
They where built from the same class, but that does not make them the same object.
If the code had been written like this:
class Department
{
public int Id { get; set; }
public string Name { get; set; }
}
Department dept1 = new Department() { Id = 1004, Name = "R&D" };
Department dept2 = dept1;
dept1.Equals(dept2);
Then the answer would be True
as they now literally hold the same object. And changing a property in one of them would also affect the other.