Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial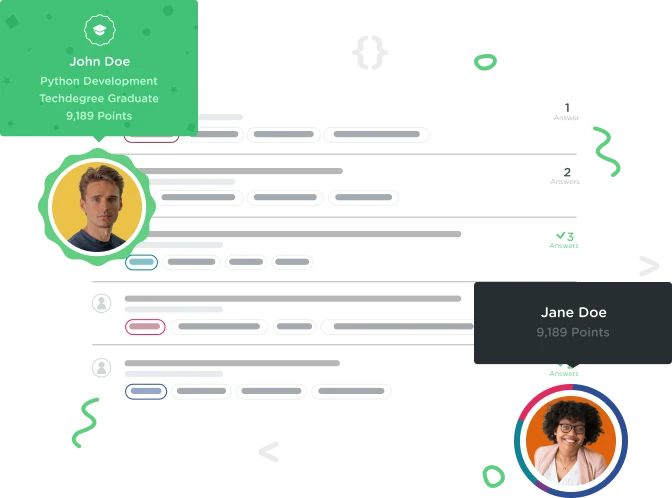
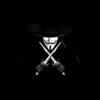
Sky Lu
1,260 PointsWhy a = [], b = [], it returns different results ?
when we coding below
a = [] b = []
a == b true
a is b false
I didn't get it about the difference between " == " and " is ". Although it mentioned in the Teacher's Notes is means A has the same memory address as B
but when you try
a = '' (Empty string)
b = ''
a == b true
a is b true
Why empty list a is b returned false?
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Sky,
When you assign empty lists to a and b you would expect those lists to be independent of each other. If I added some numbers to the a list I wouldn't expect those to show up in the b list. The b list should still be empty. This is why a and b each have their own copy of an empty list in memory. Essentially, there are 2 different locations in memory storing an empty list. a points to one and b points to the other one.
a is b
evaluates to false because those 2 objects are not the same.
The is
operator is checking for object identity. It determines this with the id()
function which will give back the id for that object. If 2 objects have the same id then they are considered to be the same object.
Here's some python output for the empty list example.
>>> a = []
>>> b = []
>>> id(a)
25690640
>>> id(b)
25692040
>>> a is b
False
>>>
Notice that the id's for the 2 objects are not the same, meaning they are not the same object. Their values at this very moment happen to be the same. The is
operator is checking if those id's are the same and it returns false because they are not.
Doing it again with empty strings:
>>> a = ""
>>> b = ""
>>> id(a)
16889952
>>> id(b)
16889952
>>> a is b
True
>>>
Here the id's are the same and so a is b
returns True. This means that there is only 1 copy of that empty string in memory which is shared by both a
and b
. Python doesn't have to do this but it can because strings are immutable. Since strings can't be changed there is no concern of updating the string at a
and having it carry over to b
This was a concern for lists because they are mutable (can be changed).
Python does this sharing with certain strings and certain numbers to save on memory.
So whenever you need to know if 2 objects are the same you would use the is
operator.
If you need to know whether 2 values are equal, you would use the ==
operator.
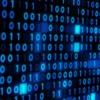
Alexander Davison
65,469 Pointsis: A has the same memory address as B
==: A is equal to B
A and B don't always have the same memory address.
They may be equal, but they don't always point to the same address in the memory.
Strings always point to the same location in the memory if the string is the same the other.
Lists don't.
I hope you understand a little more now :)

Jason Anello
Courses Plus Student 94,610 PointsHi Alexander,
I checked up on this and it's not true for all strings. This sharing of strings in python is called interning. If a string is interned then it will be shared. Not all strings are automatically interned but you can force it with intern()
For instance, a string with 20 a's will automatically be interned but a string with 21 a's will not.
>>> a = "a" * 20
>>> b = "a" * 20
>>> a is b
True
>>> a = "a" * 21
>>> b = "a" * 21
>>> a is b
False
>>>
>>> import sys
>>> a = sys.intern("a" * 21)
>>> b = sys.intern("a" * 21)
>>> a is b
True
>>>
intern()
forces the string of 21 a's to be added to the internal intern table and then a
and b
would share the same string.
It gets a bit involved on which strings are interned and which are not. Here's a blog post on the subject: http://guilload.com/python-string-interning/
Python also does this with the integers in the range -5 to 256 inclusive. Assigning integers in that range will be shared and there will be 2 copies for integers outside that range.
>>> a = 256
>>> b = 256
>>> a is b
True
>>> a = 257
>>> b = 257
>>> a is b
False
>>>
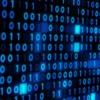
Alexander Davison
65,469 PointsOh cool! I didn't know that :)
Thanks!

youssef moustahib
2,303 Pointsthe answers for this question are waaaaay to advanced for me.. I'm going to slowly back out of here and get back to some learning..
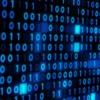
Alexander Davison
65,469 PointsDon't worry; this is confusing for many new people learning programming :)
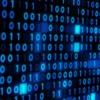
Alexander Davison
65,469 PointsActually, when you understand, it isn't so hard.
Let's pretend we have two variables called a
and the other b
. That' simple, right?
They point to some location in the computer memory. They are in the memory because we have to use the variables later. That's the whole point of variables, to store them for later access!
The "location is memory" is called the memory address. Don't worry if this is confusing; again, you don't have to read this answer if you don't feel like it.
What I was saying in my post is that strings don't usually point to the same location in memory; even if there's the exact same content in the string.
However, with lists, if they got the same content, usually they point to the same memory address.
Understand now?
Hope it does
Sky Lu
1,260 PointsSky Lu
1,260 PointsHi Jason, You are amazing, thank you very much for your reply. that's really helpful. very appreciate about that.
Michael Rathbun
1,624 PointsMichael Rathbun
1,624 PointsWhat if list a and list b ARE the exact same thing ?
For Example :
but if I do this.
In this case wouldn't list A and list B both be equivalent ?
Are you saying that no matter if whats IN THE LIST is identical (via my b=a statement) it will still be in a different memory location thus the lists can equal each other but at the same time never be truly the same thing ? Kind of like identical twins ?
Also if it has to do with memory storage are we to assume when we make certain integers equivalent to one another that memory is saved because they all align upon the same memory location ?
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Michael,
In your first code example, you end up creating 2 lists in memory. They happen to contain all the same values at this moment.
a
is a reference to one of those lists in memory andb
is a reference to the other one. You have 2 separate lists in memory that can each be changed independently from the other.This is why
a is b
returnsFalse
. It's checking the identities of those 2 variables and finding that they are not referring to the same location in memory.Your second example starts off the same way. But when you do
b = a
, you're saying thatb
is not going to reference whatevera
is referencing. That means bothb
anda
are now referring to the first 1, 2, 3 list that was originally assigned toa
.That second 1, 2, 3 list that was originally assigned to
b
no longer has any variables referencing it. In other words, there's no way to get to that list anymore.This is why
b is a
now returnsTrue
.a
andb
both have the same identities. You can think of them as aliases if that helps. It's just 2 different names for the same location in memory.Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsAlso, check my comment to Alexander's answer and see if that answers your question about integers.