Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial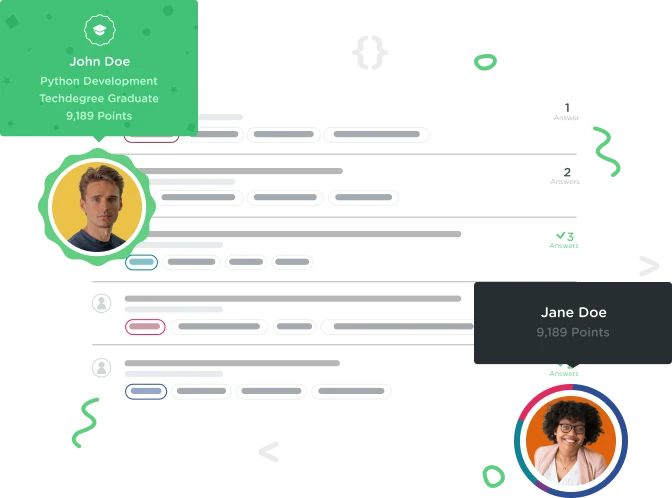
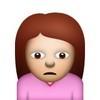
f lsze
8,021 PointsWhy "age && age >= 18" instead of just "age >=18" ?
I understand how in many cases it's important to check if a value exists before trying to do anything with it... and at 6:08 in this video Mr. Chalkley says:
If the number represents an age, and is greater than or equal to 18, we want to return
true
. If no number is passed into the function, or the number is less than 18 we want it to returnfalse
.
This is accomplished with the original function:
function isAdult( age ){
if( age && age >= 18){
return true;
}else{
return false;
}
}
console.log( isAdult(33) ); // Returns True
console.log( isAdult(9) ); // Returns False
console.log( isAdult() ); // Returns False
However in the improved function this behavior is changed and instead, when no number is passed it returns undefined
;
function isAdult( age ) {
return age && age >= 18;
}
console.log( isAdult(33) ); // Returns True
console.log( isAdult(9) ); // Returns False
console.log( isAdult() ); // Returns Undefined
My Question
Is it better to have the function return undefined
rather than false
? Or in a real world situation would return age >= 18;
be completely fine? (Which would return false
if no value is passed, as in the originally stated plan.)
1 Answer

Dale Bailey
20,269 PointsUndefined would be better as it will show you that there is a problem in your code, if no age is entered and it returns false your code will continue without showing an error. In a more real world scenario functions can be more complex and even call other functions which means you may get an error further down the line but not know where it came from making it harder to debug. In other programming languages you can enforce types and interfaces that need to be met before calling/using code, javascript is very relaxed in this sense and it can be easy to make problems for yourself if you don't check arguments.
Remember, computers are dumb and only do what you tell them to.
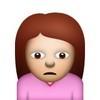
f lsze
8,021 PointsThanks I appreciate the answer. Very cool. I suppose I was thrown off because I imagined in a larger program I'd have separate handling checking if the age
has a value before even running isAdult(age)
. Although even so, I now see that it's definitely better to have the function return a separate value that I could catch or test for if age
is not set.
egaratkaoroprat
16,630 Pointsegaratkaoroprat
16,630 PointsIn some cases it might be better when the function returns undefined. It might help you find bugs in your code. When the function returns either true or false, you can never make sure whether 'age' has been assigned a value or not.
I hope this helps.