Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial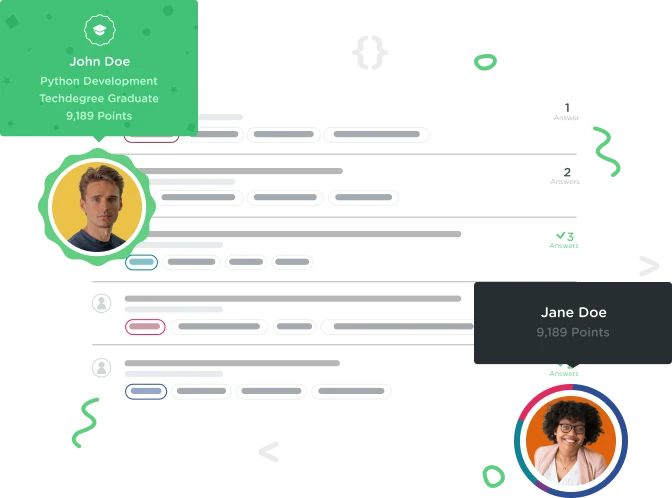

Susan Rusie
10,671 PointsWhy am I getting "404" instead of "200"?
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//Require https module
const https = require('https');
const username = "chalkers";
// Function to print message to console
function printMessage(username, badgeCount, points) {
const message = '${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript';
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get('https://teamtreehouse.com/${username}.json', response => {
console.log(response.statusCode);
// Read the data
// Parse the data
//Print the data
});

Susan Rusie
10,671 PointsThank you, Chris. Making that change to the back ticks fixed the problem.
1 Answer

Daron Anderson
2,567 PointsSo apparently mines was not working because I used my name, but not the correct username the way the URL formatted the name. So if anyone is using their name, copy and paste your username from the URL into https://teamtreehouse.com/yourusername const username = "yourusername"
i was formatting it incorrectly. didn't make sure the name in the URL was the name in the username variable.
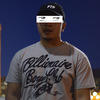
Bill Timber
3,463 PointsThank you for this!
Chris Gaona
Full Stack JavaScript Techdegree Graduate 31,350 PointsChris Gaona
Full Stack JavaScript Techdegree Graduate 31,350 PointsIt looks like you're using regular single quotes for your url string and your other strings, but you are trying to use es6 template strings. You should be using backticks, which should be right next to the esc key on your keyboard instead. You're using this:
'https://teamtreehouse.com/${username}.json'
When you should be using this:
`https://teamtreehouse.com/${username}.json`
See this link for more details: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals. Hopefully that helps!