Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial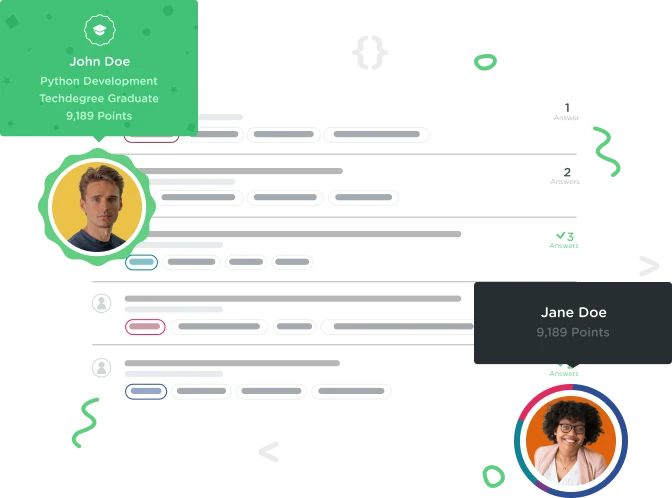

Hermes Crespo
980 PointsWhy am I getting a compiler error on my code if the method Square is indeed defined??
Why am I getting a compiler error on my code if the method Square is indeed defined??
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double Area
{
get
{
double area;
Square mySquare = new Square(4);
return area = mySquare.SideLength * mySquare.SideLength;
}
}
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Polygon
{
public int NumSides { get; private set; }
public Polygon(int numSides)
{
NumSides = numSides;
}
}
}
3 Answers

Hermes Crespo
980 PointsThanks Allen. Worked like a charm!!
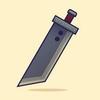
Allan Clark
10,810 PointsYou seem to be missing one of the given properties.
public double SideLength { get; private set; }
Aside from that, with a computed property it acts like a normal variable on the object, and has access to the object's properties. So you do not need to create a new square inside the computed property since you will be working with the square it is attached to. Also you can return the value directly, eliminating the need for the 'area' place holder variable. So this will change your property to look like this:
public double Area
{
get
{
return SideLength * SideLength;
}
}

Hermes Crespo
980 PointsThank you but I am still getting compiler errors. Something about the class not having a constructor with 0 parameters and then when I tried to fix it I got such one compiler error stating: "... unexpected character }..." Here is my code...
namespace Treehouse.CodeChallenges { class Square : Polygon { public double SideLength { get; private set; }
public double Area()
{
get
{
return SideLength * SideLength;
}
}
}
}
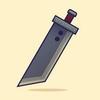
Allan Clark
10,810 PointsTake out the '()'. Only methods need the parenthesis so the compiler is trying to interpret that as a method.

Hermes Crespo
980 PointsIf I do what Allan Clark suggests I get this error:
Square.cs(3,11): error CS1729: The type Treehouse.CodeChallenges.Polygon' does not contain a constructor that takes
0' arguments
Polygon.cs(7,16): (Location of the symbol related to previous error)
Compilation failed: 1 error(s), 0 warnings
Here is the code:
namespace Treehouse.CodeChallenges { class Square : Polygon { public double SideLength { get; private set; }
public double Area
{
get
{
return SideLength * SideLength;
}
}
}
}
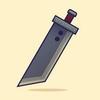
Allan Clark
10,810 Pointssounds like the call to base() got removed. Here is what the final Square Class should look like
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double SideLength { get; private set; }
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
public double Area
{
get
{
return SideLength * SideLength;
}
}
}
}
side note click 'Markdown Cheatsheet' to see how to format code to be easier to read