Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial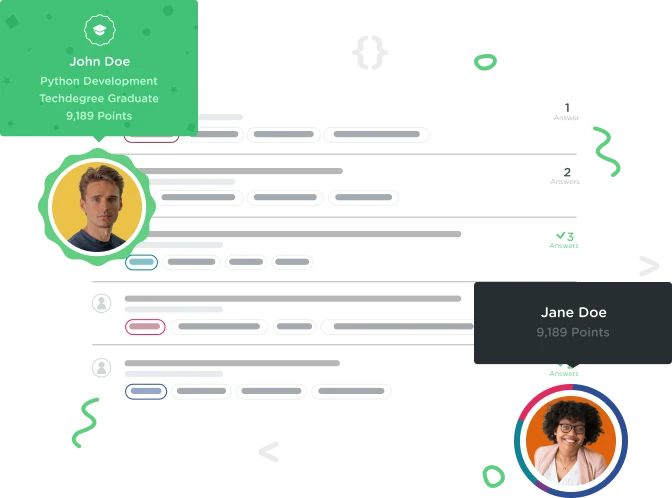

David Jones
1,681 PointsWhy am I getting a compiler error saying that my charAt method parameter should be a variable instead of a value?
I am not sure why I am getting a compiler error for the first part of the problem. Also I do not have a good idea where to start for the second part. I know we covered how to make a string or character lower case but I can't think of a good way based on what was covered so far to identify if a character in a string is upper case. The best I can think of is
boolean ex2 = (fieldName.charAt(1) = ('A' || 'B' || 'C' etc...)); if (!ex2) { throw new IllegalArgumentException ("The second letter needs to be capitalized"); }
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
boolean ex1 = (fieldName.charAt(0)= 'm');
if (!ex1) {
throw new IllegalArgumentException("field Name must start with the letter m.");
}
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
1 Answer

Craig Lovell
5,752 PointsThis wont make all of the errors go away, but the one that you are asking about is most likely caused by the line:
boolean ex1 = (fieldName.charAt(0)= 'm');
Remember, there are different operators for equality and assignment.
David Jones
1,681 PointsDavid Jones
1,681 PointsThanks for the reminder. Bellow is the second half of the problem solved. Would you have done it a cleaner or different way?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) { boolean exOne = fieldName.charAt(0)=='m'; if (!exOne) { throw new IllegalArgumentException("First letter should start with m"); } char charTwo = fieldName.charAt(1); boolean exTwo = Character.isLetter(charTwo); if(!exTwo) { throw new IllegalArgumentException("The second character should be a letter"); }
}
}
Craig Lovell
5,752 PointsCraig Lovell
5,752 PointsFor what the question is, I think your answer is perfectly fine. Of course, you will always have developers that argue about what the best way to do something is. Some might say that there is not point in creating a boolean variable like exOne, exTwo, or exThree if the only thing you are using it for is an if statement comparison. But others would argue that it provides clarity, and improves readability. But again, the other side would come right back and argue that comments would serve and equal purpose.
Writing code is often is constant battle of these various schools of thought. I used to worry about which one was correct, but eventually I learned to just write my code and not worry so much about it. If you are working on a team, then you should probably work out a common style between yourself and the other members for consistency sake. However, if it code written for only yourself, then I recommend you just write whatever makes most sense to you. There is no one perfect style. They all have pros and cons. The trick is finding the one that you will be most productive with.
Good luck.