Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial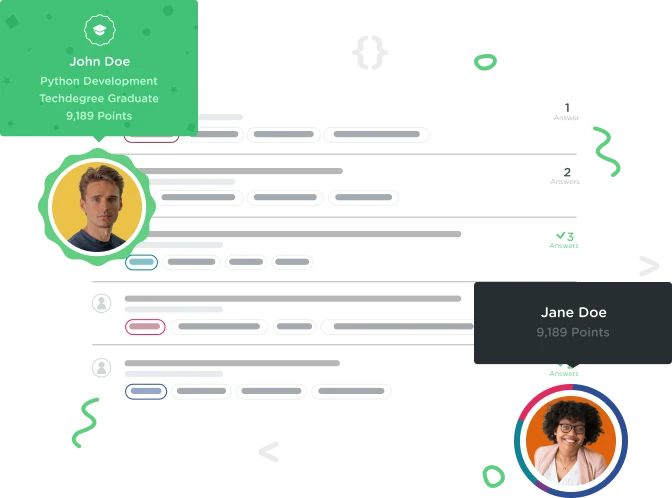
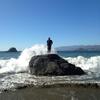
Joe Williams
4,014 PointsWhy am I getting an undefined value or when I try to check the value of my variable after submitting text from an input?
I have spent the last 3 hours trying to figure this out on my own, and I've finally hit a point where I'm absolutely stuck.
This is all I'm trying to do: I was having a hard time following along with one of the intermediate JavaScript videos, so I wanted to just play around and see if I was getting some of the concepts.
My goal was to simply create a text field and a button, and when the button was clicked, whatever was typed into the field would be saved as a variable. I wanted to be able to then goto in the console and look up the value of the variable and have it display. My mindset being, how else would I save information typed into a text box on my page and have it saved?
I ended up getting close, then breaking it, getting close, then breaking it ... and in my quest to Google, got confused by if I was to onsubmit="return false", and blah blah blah. HELP!
Below I posted my code from when I was closest to getting it right (I think):
If I brought up the console, I could type a word into the text-field and click submit and whatever I typed in would appear in the console. However, the word also remained in the search box. I then noticed if I deleted 'onsubmit="return false"' that the word would no longer appear after I clicked submit, but I also could no longer bring up the variable through the console.
Here is my html (again, it's real simple):
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="test.css">
</head>
<body>
<form onsubmit="return false">
<input id="text-1" type="text">
<input id="button-1" type="submit">
</form>
<script type="text/javascript" src="learning.js"></script>
</body>
</html>
Here is my Javascript:
var textAnswer = document.getElementById("text-1");
var submitButton = document.getElementById("button-1");
var submitInformation = function(){
console.log(textAnswer.value);
}
submitButton.onclick = submitInformation;
I had 50 other things, and as I said, seemed to only get further and further from what I was trying to do.
What am I doing wrong?
2 Answers
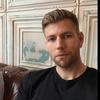
Paul Yabsley
46,713 PointsLooks like you're missing a closing curly brace on your submitInformation function:
var textAnswer = document.getElementById("text-1");
var submitButton = document.getElementById("button-1");
var submitInformation = function(){
console.log(textAnswer.value);
} // <-- missing closing brace
submitButton.onclick = submitInformation;
Try that. It logs the value of the input to the console. Is that what you were trying to do. Here are the MDN docs about functions if it helps: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/function

Lin Peng
2,251 PointsHow can I return the value "foo" from the submitInformation function ?
<script>
var textAnswer = document.getElementById("text-1");
var submitButton = document.getElementById("button-1");
var foo;
var submitInformation = function(){
foo = textAnswer.value; // Assign input value to foo
console.log(foo);
textAnswer.value = ''; // Set textAnswer value to empty string
}
submitButton.onclick = submitInformation;
</script>
Joe Williams
4,014 PointsJoe Williams
4,014 PointsI imagine that was just a copy-and-paste error, but I'll give it a shot! The console.log worked fine, which is why I'm assuming it was a typo on my part. The problem I was having was that whatever I typed into the text field didn't disappear after I clicked submit unless I used return false (which I found on Google.) Without it, the text goes away after I submit but the value was no longer saved in the variable. If I tried to find the value in the console, it'd simply say no value.
Update: Yep, typo on my part. I had the closing bracket in my code. Thanks for the attempt, though.
Paul Yabsley
46,713 PointsPaul Yabsley
46,713 PointsAh ok, well can't you just assign textAnswer a different value inside your function?
text.Answer.value = ""; // an empty string
Doing that should clear out the value in the input but you also want that value assigned to a variable which you can then use.
foo = textAnswer.value;
So if you type foo into your console initially it will be an empty string. If you submit something it will be whatever you submit.
I think there are scope issues with the above because setting foo to textAnswer.value within submitInformation means its global. You might want to be careful with that but for the purpose of what you're trying to do it demonstrates it.
Joe Williams
4,014 PointsJoe Williams
4,014 PointsThis is what I added:
But this is what I got in my console = ReferenceError: text is not defined.
If I type in what you suggested, using the variable foo, it prints to the console but gives me the same error: ReferenceError: text is not defined. Specifically, it's giving the error on having an empty value.
If I remove onsubmit="return false" from the html form, then as soon as I click submit, if I'm viewing the console, it just clears out immediately βΒ and won't bring up the variable I called.
Paul Yabsley
46,713 PointsPaul Yabsley
46,713 PointsYou have a typo above I think. Should be textAnswer.value = "" as opposed to text.Answer.
Here is a codepen of it working in the way you tried http://codepen.io/anon/pen/GgvJVg?editors=101
Joe Williams
4,014 PointsJoe Williams
4,014 PointsThat's very helpful!
This is the only issue I'm having: With the Codepen, I see that whatever I type in is appearing in the console. But if I then try to bring up textAnswer.value in the console, it's saying there's no value.
And if I take that exact HTML and JS as it appears on Codepen and put it into my text editor, it'll clear the text field, but the word quickly appears in the console then disappears again, and if you type in textAnswer.value in the console, it again says no value. (Or does Codepen not allow you to do that?)
This is a bit disheartening, because I just don't feel it should be so hard for me to create a text box, click a button, and save that info into a variable to use later on. I don't know what I'm missing.
Paul Yabsley
46,713 PointsPaul Yabsley
46,713 PointsYeah I see what you mean. I think codepen might be restricting access to the js through the console.
In my example above I didn't include the onsubmit="return false" attribute/value on form element which would mean running that locally would cause a page reload which in turn causes any variables previously set to lose their values. I'm sorry if i've confused things.
I've tried running this locally and it seems to work. It logs the input value to the console but also makes it accessible by typing foo into the console.
Paul Yabsley
46,713 PointsPaul Yabsley
46,713 PointsHere is a slightly different version that doesn't need the onsubmit attribute on the form element. Instead the page refresh is stopped by preventDefault() and stopProgragation() which are called inside the submitInformation function.
Also, instead of attaching an onclick event to the submit button there is an event listener for when the form is submitted.