Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial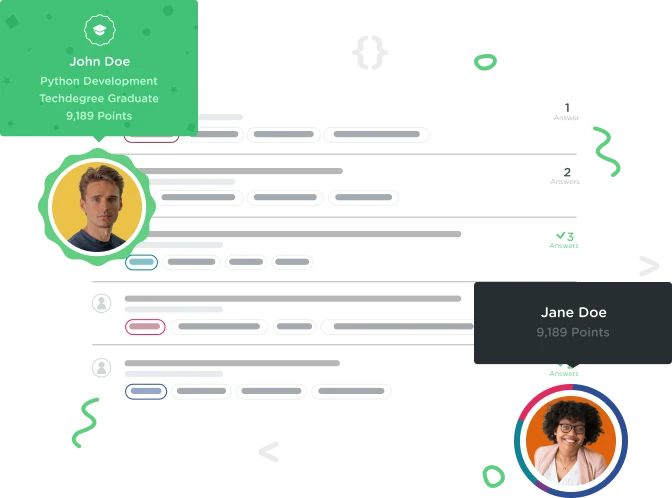

Susan Rusie
10,671 PointsWhy am I getting "cannot GET/ cards" when I go to "localhost/cards?" What am I missing?
Here is my code:
app.js
const express = require('express');
const app = express();
app.set('view engine', 'pug');
app.get('/', (req, res) => {
res.render('index');
});
app.get('/hello', (req, res) => {
res.send('<h1>Hello, JavaScript Developer!</h1>');
});
app.listen(3000, () => {
console.log('The application is running on localhost:3000!')
});
card.pug
extends layout.pug
block content
section#content
h2= prompt
if hint
p
i Hint: #{hint}
layout.pug
doctype html
html(lang="en")
head
title Flash Cards
body
include includes/header.pug
block content
include includes/footer.pug
index.pug
extends layout.pug
block content
section#content
h2 Welcome, student!
header.pug
header
h1 Flash Cards
footer.pug
footer
p The best study app ever created
Any help would be greatly appreciated. Thanks in advance.
3 Answers

apalm
Full Stack JavaScript Techdegree Graduate 27,641 PointsIt looks like you've got the card.pug file, but have left out the route wiring express to that file. If you add
app.get('/card', (req, res) => {
res.render('card');
});
to the app.js, it should work.
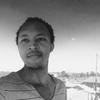
Dan Weru
48,030 PointsYou do not have a cards.pug file. Instead you have a card.pug file.

Susan Rusie
10,671 PointsI changed my "card.pug" file to "cards.pug" and I am still getting the same error. Any other suggestions on what to try?
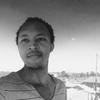
Dan Weru
48,030 PointsI’m not that comfortable with express routing, but I suppose you need to have a route for serving the pages. You could have a specific get route for the /cards path, or you could have general route that matches a pattern.

Jose Vazquez
Full Stack JavaScript Techdegree Student 12,780 PointsMaybe you just need to try "localhost:3000/cards"
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI also realized that somewhere along the way I didn't update my app.js file. It should have look like this:
I have corrected it and now everything works as it should. Thank you for your help. It was greatly appreciated.