Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial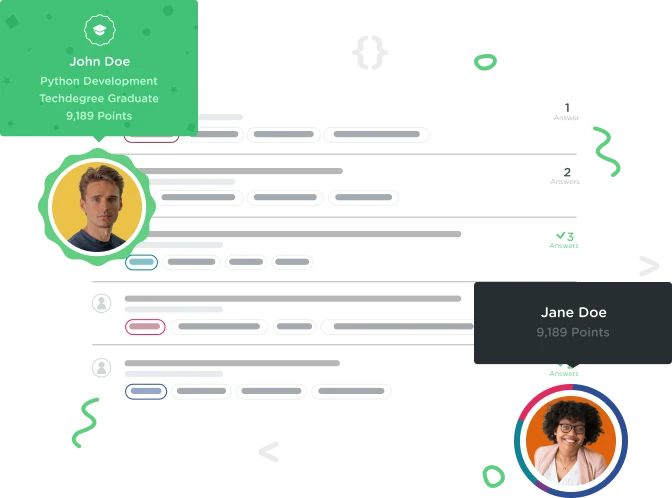

Susan Rusie
10,671 PointsWhy am I getting this error in the console?
This is the error I am getting in the console and I can't figure out why.
Rusie@DESKTOP-05UMA67 MINGW64 ~/Documents/susan_treehouse/Building_a_Rest_API/Building_the_Question_Routes (master)
$ node app.js
module.js:471
throw err;
^
Error: Cannot find module './routes'
at Function.Module._resolveFilename (module.js:469:15)
at Function.Module._load (module.js:417:25)
at Module.require (module.js:497:17)
at require (internal/module.js:20:19)
at Object.<anonymous> (C:\Users\Rusie\Documents\susan_treehouse\Building_a_R
est_API\Building_the_Question_Routes\app.js:5:14)
at Module._compile (module.js:570:32)
at Object.Module._extensions..js (module.js:579:10)
at Module.load (module.js:487:32)
at tryModuleLoad (module.js:446:12)
at Function.Module._load (module.js:438:3)
Here is my code starting with route.js:
'use strict';
var express = require("express");
var router = express.Router();
// GET /questions
// Route for questions collection
router.get("/", function(req, res){
res.json({response: "You sent me a GET request"});
});
// POST /questions
// Route for creating questions
router.post("/", function(req, res){
res.json({
response: "You sent me a POST request",
body: req.body
});
});
// GET /questions/:id
// Route for specific questions
router.get("/:id", function(req, res){
res.json({
response: "You sent me a GET request for ID " + req.params.id
});
});
module.exports = router;
My app.js
'use strict';
var express = require("express");
var app = express();
var routes = require("./routes");
var jsonParser = require("body-parser").json;
app.use(jsonParser());
app.use("/questions", routes);
var port = process.env.PORT || 3000;
app.listen(port, function(){
console.log("Express server is listening on port", port);
});
Any help on this would be greatly appreciated. Thanks in advance.

nico dev
20,364 PointsHi Susan Rusie ,
That might probably have happened if, in your Postman, when you typed the body to be sent in the POST request, you did not select (in the options above) the JSON (application/json)
radio button.
The default is Text, and when I forgot to set it appropriately (despite the fact it was mentioned before in the course), I got that exact situation: the body appeared like an empty object.
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsI realized I had left the "s" off of the route.js folder to make it routes.js so now Node works. However, that doesn't explain why when I asked for a POST request that instead of getting "color": "blue", I got this:
What did I miss to cause this to happen?