Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial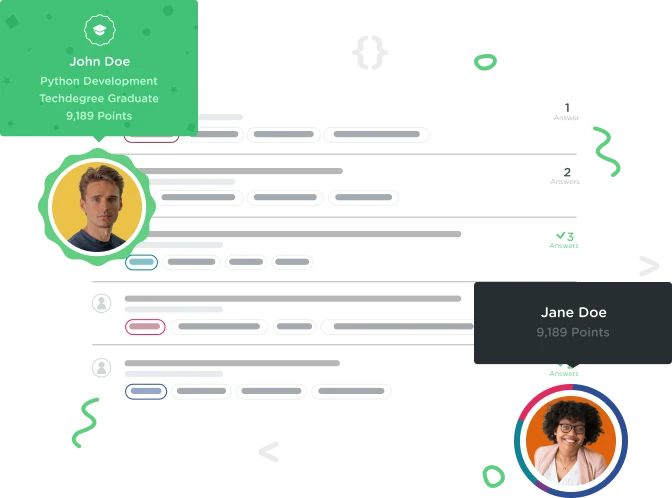

Kevin Nguyen
77 PointsWhy am I not getting my new messages?
When I swipe I am not displayed with new messages.
package com.thegreathoudini.kevin.grapevine;
import android.app.AlertDialog;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.ListFragment;
import android.support.v4.widget.SwipeRefreshLayout;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.ProgressBar;
import android.widget.Toast;
import com.parse.FindCallback;
import com.parse.Parse;
import com.parse.ParseException;
import com.parse.ParseFile;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseUser;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Kevin on 5/29/2015.
*/
public class InboxFragment extends ListFragment {
protected List<ParseObject> mMessages;
protected SwipeRefreshLayout mSwipeRefreshLayout;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_inbox, container, false);
mSwipeRefreshLayout = (SwipeRefreshLayout)rootView.findViewById(R.id.swipeRefreshLayout);
mSwipeRefreshLayout.setOnRefreshListener(mOnRefreshListner);
mSwipeRefreshLayout.setColorSchemeResources(R.color.swipeRefresh1, R.color.swipeRefresh2, R.color.swipeRefresh3, R.color.swipeRefresh4);
return rootView;
}
@Override
public void onResume() {
super.onResume();
getActivity().setProgressBarIndeterminateVisibility(true);
retrieveMessages();
}
private void retrieveMessages() {
ParseQuery<ParseObject> query = new ParseQuery<ParseObject>(ParseConstants.CLASS_MESSAGES);
query.whereEqualTo(ParseConstants.KEY_RECIPIENTS_IDS , ParseUser.getCurrentUser().getObjectId());
query.orderByDescending(ParseConstants.KEY_CREATED_AT);
query.setCachePolicy(ParseQuery.CachePolicy.CACHE_ELSE_NETWORK);
query.findInBackground(new FindCallback<ParseObject>() {
@Override
public void done(List<ParseObject> messages, ParseException e) {
if(mSwipeRefreshLayout.isRefreshing()){
mSwipeRefreshLayout.setRefreshing(false);
}
if(e == null){
getActivity().setProgressBarIndeterminateVisibility(true);
mMessages = messages;
int i = 0;
String [] usernames = new String[mMessages.size()];
for (ParseObject message: mMessages){
usernames[i] = message.getString(ParseConstants.KEY_SENDER_NAME);
i++;
}
if(getListView().getAdapter() == null){
MessageAdapter adapter = new MessageAdapter(
getListView().getContext(), mMessages);
setListAdapter(adapter);
}
else{
((MessageAdapter)getListView().getAdapter()).refill(mMessages);
}
}
}
});
}
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
ParseObject message = mMessages.get(position);
String messageType = message.getString(ParseConstants.KEY_FILE_TYPE);
String messageComment = message.getString(ParseConstants.KEY_COMMENT_TEXT);
ParseFile file = message.getParseFile(ParseConstants.KEY_FILE);
Uri fileUri = Uri.parse(file.getUrl());
if(messageType.equals(ParseConstants.TYPE_IMAGE)){
//View image
Intent intent = new Intent(getActivity(), ViewImageActivity.class);
intent.setData(fileUri);
intent.putExtra(ParseConstants.KEY_COMMENT_TEXT,messageComment);
startActivity(intent);
}
}
protected SwipeRefreshLayout.OnRefreshListener mOnRefreshListner = new SwipeRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
retrieveMessages();
}
};
}
1 Answer

Kevin Nguyen
77 PointsHey Harry James Thanks for looking into it. I found it this morning. It was the cache line that I had in there before calling the query.
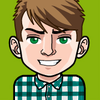
Harry James
14,780 PointsAh! Glad to hear you fixed your problem.
I was wondering what that cache line was doing - had to look it up on the Parse Javadocs!
I do like your thinking in this sense though! Do look around at all the awesome stuff Parse has because there is tons (And they are, all awesome).
If there's anything else you get stuck on at some point then please feel free to tag me again! (It helps me learn new stuff too!) :)
Harry James
14,780 PointsHarry James
14,780 PointsHey Kevin!
I'll get back to you on this after I return from work today.
Speak to you soon :)