Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial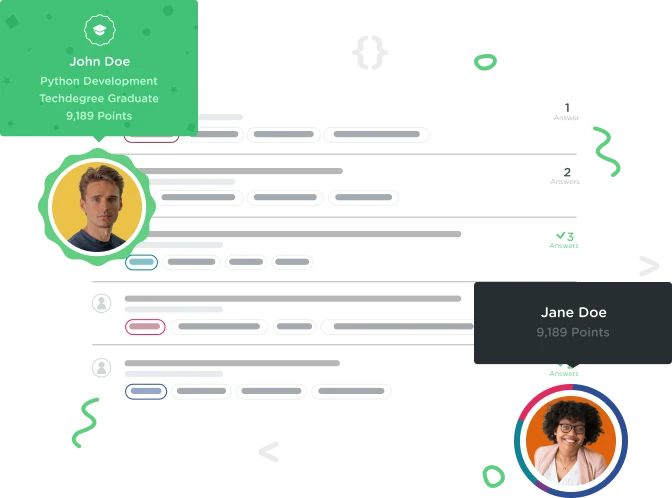
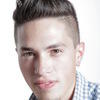
Sam Alpren
4,744 PointsWhy am I not getting random colors for the parameters in my second function 'randomColor2'?
The colors seem to repeat every time. I tried nesting another loop inside, but the parameters stay the same even when the third loop on the file runs. What is wrong with the function that it is holding the values?
1 Answer

Emmanuel C
10,636 PointsHey, the vars red, green and blue only get assigned in the first for loop. But not in the other loops. When you pass a parameter into a function in JS they get passed in by value, which basically means that a copy of the variable is made in the function and that is what is getting assign. So when you pass red into your functions the random number is being assigned to a copy of that variable, 'q' but the original red remains the same number.
If you've noticed those circles that have the same color, actually have the same color of the 10th and last circle of the of the first for loop because thats the last time the red, blue and green vars were assigned. In the other two functions only copies of those vars were assigned, not the originals.
One way to fix this is to make red, green, blue a global var at the top and just reassign them in your functions instead of using parameters. Also since your rgbColor var for the 2nd and 3rd function doesnt get assigned again either, the 10 circles that get printed in those loops would be the same color, even though the numbers are changing and are properly being assigned. So I moved them into the loops just like for the first function.
var red;
var green;
var blue;
//original
function randomColor(x) {
x = Math.floor(Math.random() * 256);
return x;
}
for (var i = 0; i < 10; i++) {
red = randomColor();
green = randomColor();
blue = randomColor();
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.write('<div style="background-color:' + rgbColor + '"></div>');
}
// attempt 2
function randomColor2() {
red = Math.floor(Math.random() * 256);
green = Math.floor(Math.random() * 256);
blue = Math.floor(Math.random() * 256);
}
for (var i = 0; i < 10; i++) {
randomColor2();
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.write('<div style="background-color:' + rgbColor + '"></div>');
}
console.log(red);
console.log(green);
console.log(blue);
//attempt 3 nested loop
function randomColor3() {
red = Math.floor(Math.random() * 256);
green = Math.floor(Math.random() * 256);
blue = Math.floor(Math.random() * 256);
}
for (var i = 0; i < 10; i++) {
for (var j = 0; j < 10; j++) {
randomColor3();
}
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
document.write('<div style="background-color:' + rgbColor + '"></div>');
}
console.log(red);
console.log(green);
console.log(blue);
Good luck man, hope this help.
Sam Alpren
4,744 PointsSam Alpren
4,744 PointsWow, thanks man! I can't believe I didn't think to notice I was just reusing the same variables for red, blue and green. This is great.