Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial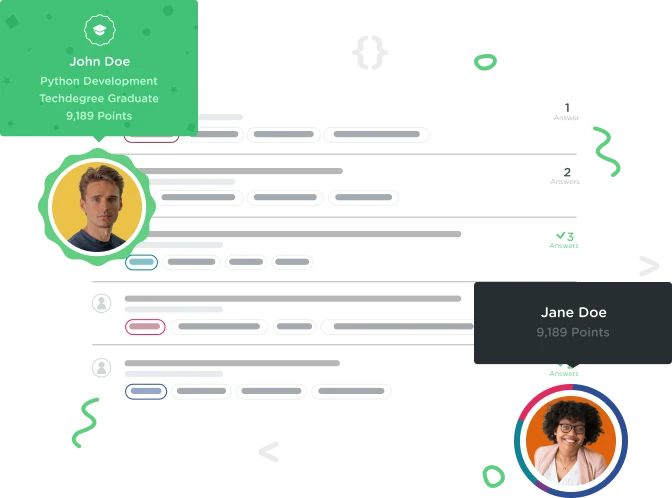

Christopher Bush
Courses Plus Student 8,382 PointsWhy am I not returning the correct index?
I know I am close, and getting frustrated. Thanks for any help.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
@todo_items.each do |todo_item|
if todo_item.name == name
found = true
end
index += 1
break if found
end
if found
return index
else
return nil
end
end
end
2 Answers
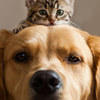
Devin Scheu
66,191 PointsYour code should look like this, you can compare what you did wrong to it:
def find_index(name)
index = 0
found = false
todo_items.each do |todo_items|
if todo_items.name == name
found = true
break
else
index += 1
end
end
if found
return index
else
return nil
end
end

Christopher Bush
Courses Plus Student 8,382 PointsThanks a bunch!!!!!!!!!