Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial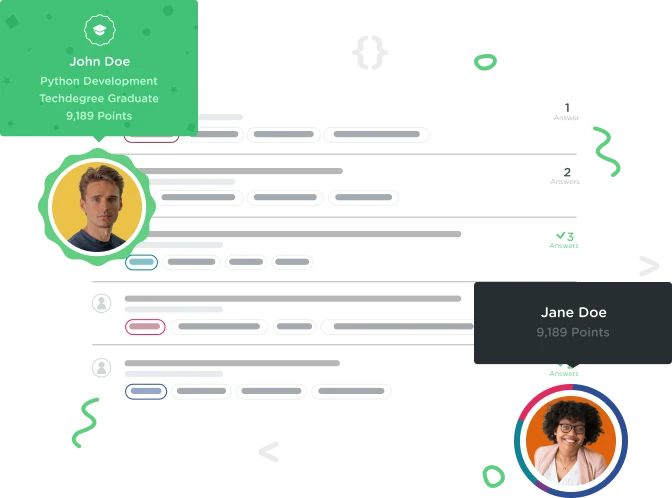
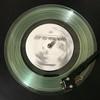
Tim Balzer
13,315 PointsWhy am I receiving an InternalError when trying to handle the IntegrityError?
Rather than working in the Workspace, I set up peewee to use my local PostgreSQL database. However, I receive an InternalError when I try to handle the IntegrityError in this exercise.
Code:
def add_students():
for student in students:
try:
Student.create(username=student["username"],
points=student["points"])
except IntegrityError:
student_record = Student.get(username=student["username"])
student_record.points = student["points"]
student_record.save()
Error:
peewee.InternalError: current transaction is aborted, commands ignored until end of transaction block
2 Answers
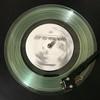
Tim Balzer
13,315 PointsThe way to get around this was to actually to rollback the transaction before continuing. Here's what ended up working for me:
def add_students():
for student in students:
try:
Student.create(username=student["username"],
points=student["points"])
except IntegrityError:
db.rollback()
student_record = Student.get(username=student["username"])
student_record.points = student["points"]
student_record.save()
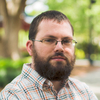
Kenneth Love
Treehouse Guest TeacherHmm, interesting. I'm guessing that the .create()
got Postgres into a weird state, but it doesn't seem like it should have.

Jack Fuller
5,859 PointsDid anyone get to the bottom of his? I've just spent an hour trying to work out why the exception didn't just catch without the rollback.
Sorry for the long post.
Traceback (most recent call last):
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 3748, in execute_sql
cursor.execute(sql, params or ())
psycopg2.IntegrityError: duplicate key value violates unique constraint "student_username"
DETAIL: Key (username)=(james) already exists.
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "student.py", line 32, in add_students
points=student['points'])
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 4889, in create
inst.save(force_insert=True)
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 5082, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 3506, in execute
cursor = self._execute()
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 2892, in _execute
return self.database.execute_sql(sql, params, self.require_commit)
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 3755, in execute_sql
self.commit()
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 3578, in __exit__
reraise(new_type, new_type(*exc_args), traceback)
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 135, in reraise
raise value.with_traceback(tb)
File "/Users/jackfuller/python/databases/databasing/lib/python3.6/site-packages/peewee.py", line 3748, in execute_sql
cursor.execute(sql, params or ())
peewee.IntegrityError: duplicate key value violates unique constraint "student_username"
DETAIL: Key (username)=(james) already exists.
The above ran twice before printing this line and exiting:
peewee.InternalError: current transaction is aborted, commands ignored until end of transaction block
Yeeka Yau
7,410 PointsYeeka Yau
7,410 PointsThanks for posting this, I am doing the same thing on my local machine with postgresql. I had the exact same problem.