Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial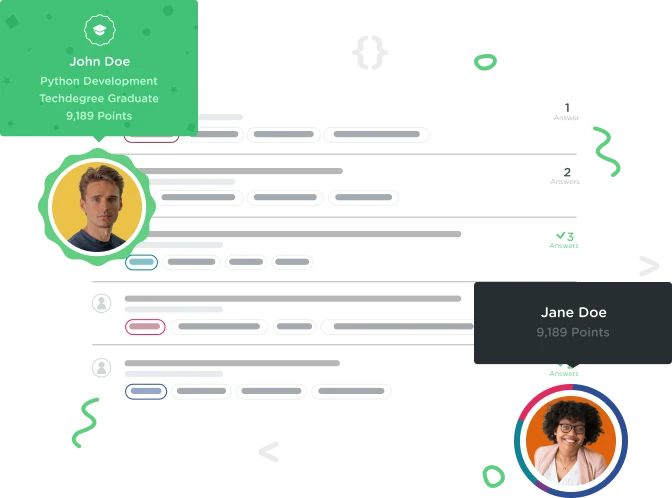

Ian Maina
4,571 Pointswhy are my tests not passing. what is wrong with my code
I need help figuring out where I went wrong in my code.
from django.db import models
# Write your models here
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
performer = models.ForeignKey("Performer")
length = models.IntegerField(default=0)
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
class Performer(models.Model):
name = models.CharField(max_length=255)
def __str__(self):
return self.name
from django.shortcuts import render
from .models import Song, Performer
def song_list(request):
songs = Song.objects.all()
return render(request, "songs/song_list.html", {"songs": songs})
def song_detail(request, pk):
song = Song.objects.get(Song, pk=pk)
return render(request, "songs/song_detail.html", {"song": song})
def performer_detail(request, song_pk, perf_pk):
performer = Performer.objects.get(Performer, song_id=song_pk, pk=perf_pk)
songs = Song.objects.filter(performer=performer)
return render(request, "songs/performer_detail.html", {"perfomer": performer}, {"songs": songs})
{% extends 'base.html' %}
{% block title %}{{ performer.name }}{% endblock %}
{% block content %}
<h2>{{ performer }}</h2>
{% for song in songs %}
{{ song }}
{% endfor %}
{% endblock %}
1 Answer

dorotevi tenmpo
30 PointsWhat is the Performer
argument in your performer
variabile inside the function performer_detail
, delete that.
Or maybe you want to use get_object_or_404.