Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial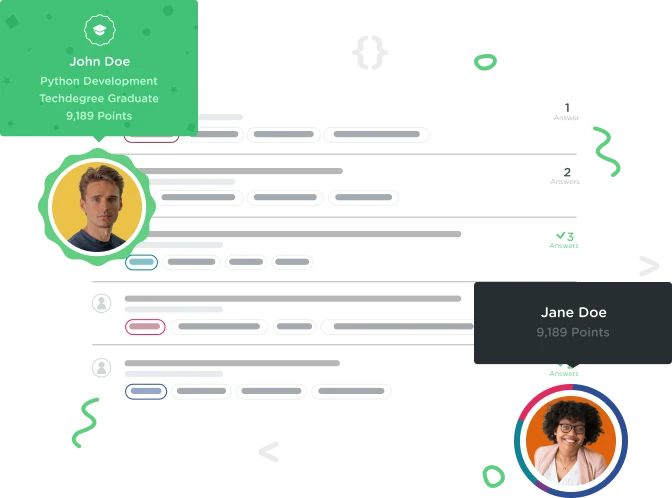

Alfredo Takori
1,818 PointsWhy are the numbers showing up as undefined? AND parseInt???
My solution for the quiz works, but the only part that does not is the amount of questions answered correctly / wrong (comes up as undetermined). Also, my second question requires the parseInt function in order to take a number as the answer, but none of my other questions are number based. How can I get parseInt for that one particular answer if I am using a loop for the quiz???
var quizQandA = [["What is the name of Alfredo's Tiger?", "timothy"], ["How many different countries has alfredo been to?", "two"], ["What instrument is Alfredo learning how to play?" , "classical guitar"]]; var response; var correct = "You got " + right + " questions correct" + "<p> Questions that you got right: </p> "; var inCorrect = "You got " + wrong + " questions incorrect" + "<p> These questions are the one you got wrong. Do better. </p> "; var right; var wrong;
function print(message) { document.write(message); }
function ask( ) { for ( var i = 0; i < quizQandA.length ; i +=1) { response = prompt(quizQandA[i][0]); response === response.toLowerCase(); if (response === quizQandA[i][1]) { correct += "<ol>" + quizQandA[i][0] + "</ol>"; right += 1; } else { inCorrect += "<ol>" + quizQandA[i][0] + "</ol>"; wrong +=1; } } }
ask(); print(correct); print(inCorrect);
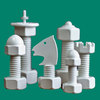
Steven Parker
231,269 PointsYou share your workspace by making a snapshot. Click on the link for instructions.
2 Answers

Benjamin Barslev Nielsen
18,958 PointsFirst parseInt is not needed, since you will not need to convert a string to a number in order to solve this exercise. I have explained below, what can be changed in your code, to make a solution that works:
The numbers are undefined since you do not assign anything to them, at first you should assign the value 0 to them.
var right = 0;
var wrong = 0;
Now you will see that 0 is printed instead. This is because you create the correct and inCorrect strings before you ask the questions, i.e., before right and wrong gets updated, and therefore it is always the value 0, that will be showed. Therefore you need to create these strings after you have asked the questions.
Therefore I will suggest introducing two new variables: correctQuestions and inCorrectQuestions, and then update these in the ask method.
Then after your call to ask, you can create the strings:
var correct = "You got " + right + " questions correct" +
"<p> Questions that you got right: </p> " +
correctQuestions;
var inCorrect = "You got " + wrong + " questions incorrect" +
"<p> These questions are the one you got wrong. Do better. </p> " +
inCorrectQuestions;
Before I wrap this up, I have a couple of comments to your ask method:
function ask( ) {
for ( var i = 0; i < quizQandA.length ; i +=1) {
response = prompt(quizQandA[i][0]);
response === response.toLowerCase();
if (response === quizQandA[i][1]) {
correctQuestions += "<ol>" + quizQandA[i][0] + "</ol>";
right += 1;
} else {
inCorrectQuestions += "<ol>" + quizQandA[i][0] + "</ol>";
wrong +=1;
}
}
}
In line 3 inside the method, it should be
response = response.toLowerCase();
since using === does not change the value of response. Besides this the HTML you create in correctQuestions and inCorrectQuestions seems weird. Recall that <ol> creates a new list, while <li> creates items inside a list. This means that you create a new list for each correctQuestion and inCorrectQuestion, instead of creating one list for correctQuestions and one for inCorrectQuestions and then appending items to these lists. The following shows how this can be fixed:
function ask( ) {
correctQuestions = "<ol>";
inCorrectQuestions = "<ol>";
for ( var i = 0; i < quizQandA.length ; i +=1) {
response = prompt(quizQandA[i][0]);
response = response.toLowerCase();
if (response === quizQandA[i][1]) {
correctQuestions += "<li>" + quizQandA[i][0] + "</li>";
right += 1;
} else {
inCorrectQuestions += "<li>" + quizQandA[i][0] + "</li>";
wrong +=1;
}
}
correctQuestions += "</ol>";
inCorrectQuestions += "</ol>";
}
Now putting these small pieces together, we should get a working solution:
var quizQandA = [["What is the name of Alfredo's Tiger?", "timothy"], ["How many different countries has alfredo been to?", "two"], ["What instrument is Alfredo learning how to play?" , "classical guitar"]];
var response;
var right = 0;
var wrong = 0;
var correctQuestions = "";
var inCorrectQuestions = "";
function print(message) { document.write(message); }
function ask( ) {
correctQuestions = "<ol>";
inCorrectQuestions = "<ol>";
for ( var i = 0; i < quizQandA.length ; i +=1) {
response = prompt(quizQandA[i][0]);
response = response.toLowerCase();
if (response === quizQandA[i][1]) {
correctQuestions += "<li>" + quizQandA[i][0] + "</li>";
right += 1;
} else {
inCorrectQuestions += "<li>" + quizQandA[i][0] + "</li>";
wrong +=1;
}
}
correctQuestions += "</ol>";
inCorrectQuestions += "</ol>";
}
ask();
var correct = "You got " + right + " questions correct" +
"<p> Questions that you got right: </p> " +
correctQuestions;
var inCorrect = "You got " + wrong + " questions incorrect" +
"<p> These questions are the one you got wrong. Do better. </p> " +
inCorrectQuestions;
print(correct);
print(inCorrect);
Hope this helps.

Alfredo Takori
1,818 Pointsthanks so much for all the help guys!
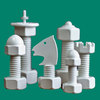
Steven Parker
231,269 PointsYou create the "correct" string using right, but you have not defined right yet. Same with "inCorrect" and wrong. You probably want to wait until you have the totals (in the ask function) before you create those strings.
Also, you don't need to convert if you just use "2" as the correct answer string.
Alfredo Takori
1,818 PointsAlfredo Takori
1,818 PointsAlso, apparently I don't know how to attach my workspace so that it is easy to read for the community. Does anyone know how to do that as well?