Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial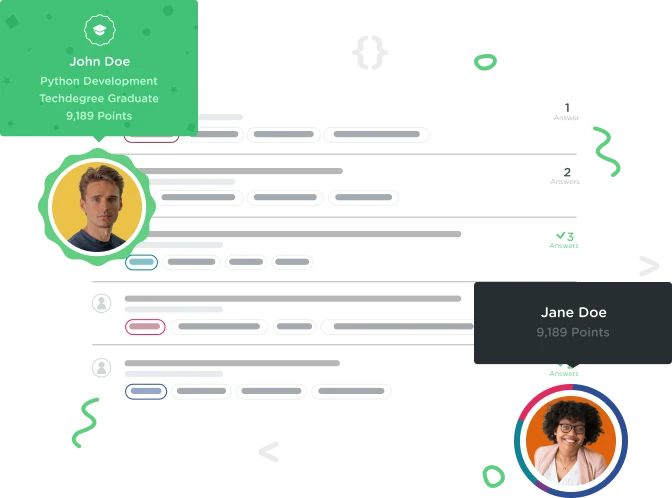

Charles Garza
652 PointsWhy are these logical conditions not working for my if-loop?
The error is reading: Bummer! Make sure you are performing logical checks in the condition of the if statement, however, I thought variables representing boolean values would be valid logical checks.
var results: [Int] = []
for n in 1...100 {
// Enter your code below
var even: Boolean = (0 == n % 2)
var isDivisible: Boolean = (0 == n % 7)
if !even && isDivisible {
results.append(n)
}
// End code
}
2 Answers

andren
28,558 PointsSeparating the conditions into variables is not strictly necessary, but you are right that doing so is a completely valid way of completing this task. The error message Treehouse gives can often be a bit misleading, it is often more helpful to look at the errors that the Swift compiler generates, which you can do by clicking on the "Preview" button after running your code.
For your code the error messages generated is this:
swift_lint.swift:10:15: error: use of undeclared type 'Boolean'
var even: Boolean = (0 == n % 2)
^~~~~~~
swift_lint.swift:11:22: error: use of undeclared type 'Boolean'
var isDivisible: Boolean = (0 == n % 7)
^~~~~~~
The thing those messages point out is that Swift does not recognize the word Boolean
as a type, that is because the type for Boolean
variables in swift is actually the word Bool
.
So if you replace Boolean
with Bool
like this:
var results: [Int] = []
for n in 1...100 {
// Enter your code below
var even: Bool = (0 == n % 2)
var isDivisible: Bool = (0 == n % 7)
if !even && isDivisible {
results.append(n)
}
// End code
}
Then your code will work.
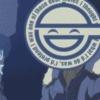
Jonathan Ruiz
2,998 PointsHi Charles this is a great way to approach this ! your very very close all you need to do is take out the part where you reference the type Boolean for the variables and it'll pass
var even = (0 == n % 2)