Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial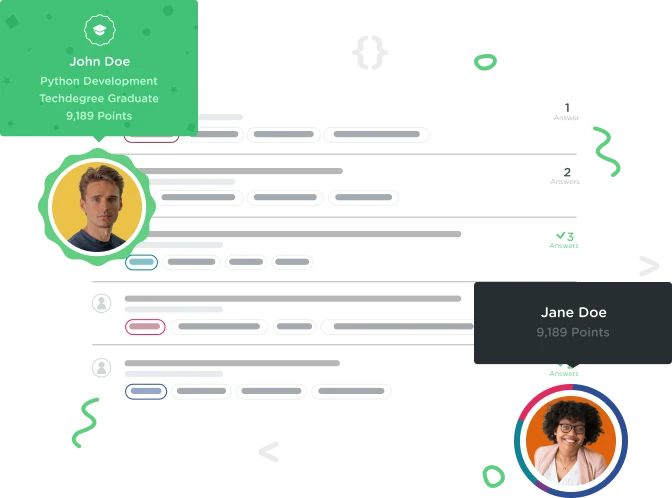
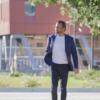
Bappy Golder
13,449 PointsWhy are we storing the HTML inside a jQuery or '$()'
Why are we storing the HTML inside a jQuery type call.
I mean why are we doing $("html here")
instead of doing "html here"
So I did this and still works just fine:
//format the information in the fields in correct HTML
var $newPet = '<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $petName +
'</p><p><strong>Species:</strong> ' + $petSpecies +
'</p><p><strong>Notes:</strong> ' + $petNotes +
'</p><span class="close">×</span></div></section>';
Wondering why Alisha put her HTML inside $()
5 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Bappy Golder ,
In this particular example, it wasn't strictly necessary to use $(). All that ends up happening is that html gets appended to the posted-pets div. The append method is able to accept an html string which is why it worked for you when you only had a string.
Also, if you do leave it as a simple string then the $newPet variable should not have a $ in front because it's no longer storing a jQuery object. It's only a simple string.
The advantage to doing it like the video is so that you can further manipulate the html with other jQuery methods.
You'll find examples of this in jQuery Basics. In particular, the light box stage.
You'll see some code like this:
var $overlay = $('<div id="overlay"></div>');
var $image = $("<img>");
var $caption = $("<p></p>");
And then later on some code like this:
$image.attr("src", imageLocation);
If $image wasn't a jQuery object then you wouldn't be able to call the jQuery .attr()
method to change the source of the image.
In a comment, you gave the example `var $test = '<p>html content</p>'. In this case as well, $test shouldn't have the dollar sign because it's not storing a jQuery object. It's not even storing a paragraph element. It's a simple string that happens to contain the html necessary to create a paragraph element.
When you do $('<p>html content</p>')
, jQuery is creating an actual paragraph element and wrapping it in a jQuery object.
Let me know if anything is still unclear.

Edward Ries
7,388 PointsI have many years of experience developing with jQuery and I can't think of any reason that you would want to wrap it the way she did. It makes no sense to me.

A M
8,369 PointsI agree.
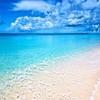
eodell
26,386 PointsThank you for the response. In the video, the instructor indicated that adding '$' before the variable name is the convention for storing jQuery objects as variables. she instantiated (<- Not sure if I'm using that word correctly, I'm still learning) 3 variables like this:
//grab info from form
var $name = $('#pet-name').val();
var $species = $('#pet-species').val();
var $notes = $('#pet-notes').val();
then she used those variables in the other code:
var $newPet = $( '<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $name + '</p><p><strong>Species:</strong> ' + $species + '</p><p><strong>Notes:</strong> ' + $notes + '</p><span class="close">Ć</span></div></section>' );
so maybe transitively, since the variables in that string are jQuery objects, the new string itself is a jQuery object? I'm not sure if that's correct or if it even makes sense. If you think I'm misunderstanding, please let me know!
Thanks.
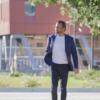
Bappy Golder
13,449 PointsHugh. that probably make scense E Gushi She is considering both side of the code as jQuery object. But don't think we need to introduce jQuey here. We just need to see them as JavaScript objects.

Jason Anello
Courses Plus Student 94,610 PointsHi E Gushi ,
The teacher correctly explained the convention but I don't think it was correctly used in the code.
$name, $species, and $notes should NOT have had dollar signs in front because they're all storing simple strings.
The .val() method with no arguments is returning a string of the text that's in the input fields.
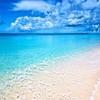
eodell
26,386 PointsSorry for the confusion, but are you wondering why she stored the new html in a jQuery object rather than vanilla JS?

Edward Ries
7,388 PointsShe put the output text inside the jQuery selector.
var $newPet = $( '<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $name + '</p><p><strong>Species:</strong> ' + $species + '</p><p><strong>Notes:</strong> ' + $notes + '</p><span class="close">Ć</span></div></section>' );
This is the line of code. I find it interesting that the newPet has a $ before it as well. I must admit, I haven't watched the video but it does seem a bit strange.
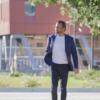
Bappy Golder
13,449 PointsYes. i.e:
Why: var $test = $('<p>html content</p>')
Rather than var $test = '<p>html content</p>'

A M
8,369 PointsI honestly think this is a very poor example of adding HTML from jQuery - it makes no logical sense, if you were actually developing the site to do that. You would just write the markup in your index file and do the JS work in your JS file. I understand that she's trying to teach new methods but ideally, in real world, you wouldn't do something like this.
Bappy Golder
13,449 PointsBappy Golder
13,449 PointsThanks Jason that makes a lot of scense. Cheers for that.