Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial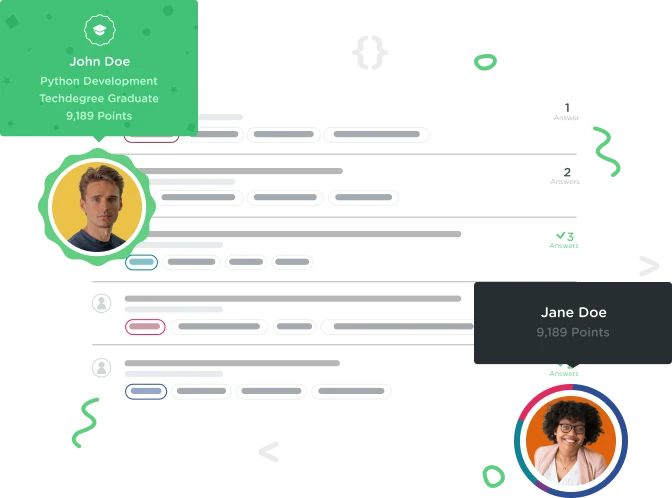

Billy Saefong
521 PointsWhy are we using return when we aren't asking for user input? IM STUCK.
I'm stuck. I don't understand why we can't simply use a WriteLine in the Eat method. Why are we using return?
using System;
class Program
{
// YOUR CODE HERE: Define an Eat method!
static string Eat(string foodOne, string foodTwo)
{
return "I think foodOne and foodTwo are tasty!";
}
static void Main(string[] args)
{
Console.WriteLine(Eat(" apples ", " blueberries "));
Console.WriteLine(Eat("carrots", "daikon"));
}
}
2 Answers
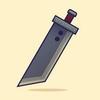
Allan Clark
10,810 PointsThe code is structured this way for educational purposes. It is meant to show you how string interpolation can be used, and it is using the return value of a separate method to show code reuse. It could certainly be structured differently as you indicated, but i believe this structure was chosen to isolate where the string interpolation takes place. Trying to cut down on the code noise where the new concept being addressed.
With that said there are a couple tweaks that need to be made to the Eat method you provided.
static string Eat(string foodOne, string foodTwo)
{
return $"I think {foodOne} and {foodTwo} are tasty!";
//the $ at the start tells the compiler that anything inside {}
//will be a variable that needs to be added to the string
}

Tom Hartley
311 Pointsusing System;
class Program
{
// YOUR CODE HERE: Define an Eat method!
static string Eat(string foodOne, string foodTwo)
{
return $"I think {foodOne} and {foodTwo} are tasty!";
// Don't print anything out in the 'Eat' Method. That is done in the 'Main Method below.
}
static void Main(string[] args)
{
Console.WriteLine(Eat("apples", "blueberries"));
Console.WriteLine(Eat("carrots", "daikon"));
}
}
Conner Davis
13,159 PointsConner Davis
13,159 PointsYou might have figured this out already, but the point is to move the work out of the main method. You could easily use two Writeline methods to perform the task, but when you move beyond simpler Console applications it'll be more clear as to why you want to move things into separate methods. Here's code for the Eat method that will work: Imgur