Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial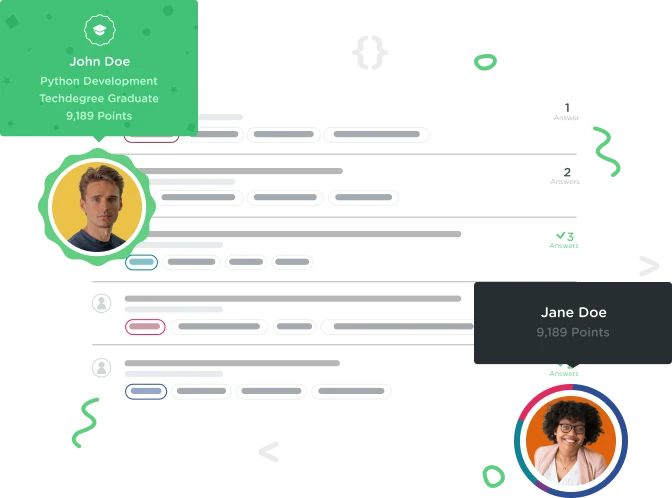

Japjot Singh
iOS Development Techdegree Student 573 PointsWhy are we using void in "public Void charge(){" is it because the variable charge has an integer value?
I ask this because earlier when we declare "public String getColor(){" we use String, I am assuming we used String because the value of the color is a string, where in the reference to the charge value it is an integer
public class GoKart { //Declare a Public Class for the Object GoKart
private String mColor; //Declare a private variable, car color as a string
public static final int MAX_ENERGY = 8; //Public but fixcd integer value for the max energy
private int mBarsCount; //Declare Private variable Bar count
public GoKart(String color) { // Declare the objects color
mColor = color; //member color is the variable
mBarsCount = 0; //fixed starting value
}
public String getColor() {
return mColor;
}
public Void charge() { //Beacuse it is a integer use void?
set mBarsCount;
}
}
2 Answers

Simon Coates
28,694 Pointscode should be:
public class GoKart { //Declare a Public Class for the Object GoKart
private String mColor; //Declare a private variable, car color as a string
public static final int MAX_ENERGY = 8; //Public but fixcd integer value for the max energy
private int mBarsCount; //Declare Private variable Bar count
public GoKart(String color) { // Declare the objects color
mColor = color; //member color is the variable
mBarsCount = 0; //fixed starting value
}
public String getColor() {
return mColor;
}
public void charge() { //Beacuse it is a integer use void?
mBarsCount = MAX_ENERGY;
}
}
void in method declaration means that nothing is returned from that method.
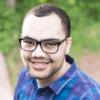
Philip Gales
15,193 PointsTo understand why we use "void" requires us to better understand methods and their signatures.
The first line of a method is called a method signature. In your case, the method signature is
public void charge()
The three parts that make up this signature are
- "public". We can use public (any other class or method can use this method), or private (only methods inside of our class can use this method)
- "void". Methods can perform a function, and maybe write to the console. Or, they can return something back to the place that called the method. By using void you are saying you only want the method to perform a function, and maybe print to the console. Below I demonstrate returning an int.
- "charge()". The method name, charge() is the last part of a method signature.
public static void main(String[] args) {
//here I declare an int x
int x;
//here i assign the variable x to the returned value of add()
x = add(3, 6);
console.print(x);
}
public int add(int firstNumber, int secondNumber) {
//because I used "int" and not void in the method signature I must have a return
//statement like the one below. This will return a number (the 2 numbers added)
//and in the main method above it will be assigned to x
return firstNumber + secondNumber;
}
The console will then print out "9", because 3 + 6 = 9. If I tried doing this with a void in the method signature I would get an error.