Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial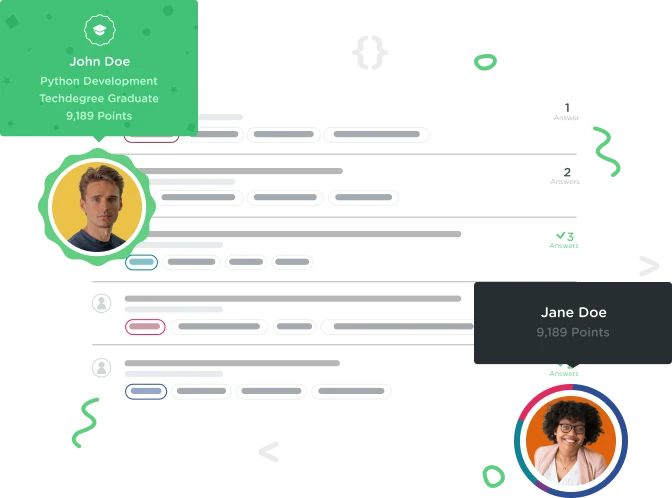

Erika Reiman
4,529 PointsWhy aren't all my players rendering in the DOM? I have added multiple Player components but only one player shows up
const Header = (props) => { return ( <header> <h1>{ props.title }</h1> <span className = "stats">Players: { props.totalPlayers }</span> </header> ); }
const Player = (props) => { return ( <div className = "player"> <span className = "player-name"> {props.name} </span> <Counter score={props.score}/> </div>
);
}
const Counter = (props) => { return ( <div className="counter"> <button className="counter-action decrement"> - </button> <span className="counter-score">{ props.score }</span> <button className="counter-action increment"> + </button> </div> ); }
const App = () => { return ( <div className="scoreboard"> <Header title="Scoreboard" totalPlayers = {1} /> {/* Players list */} <Player name="Guil" score={50} /> <Player name="Treasure" score={90} /> <Player name="Ashley" score={85} /> <Player name="James" score={80} /> </div> ); }
ReactDOM.render( <App />, document.getElementById('root') );

Erika Reiman
4,529 PointsThanks Kris! I am actually not using a workspace but attempting to use VSCode and a SimpleHTTPServer. Would screenshots of those be helpful?

KRIS NIKOLAISEN
54,972 PointsScreenshots are hard to work with. Can you paste your code for index.html? I see a lot of errors in the console using the video's workspace.
2 Answers

Yasir alabadi
5,744 PointsDid you figure this out? From what I can see the code seems fine to me., but hard to tell, it would beneficial for all parties if you are to either screenshot your code, of paste it according to the guidelines so that we can help you better :)

Erika Reiman
4,529 PointsThis is my index.html code:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Scoreboard</title> <link rel="stylesheet" href="./app.css" /> </head>
<body> <div id="root"></div> <script crossorigin src="https://unpkg.com/react@16/umd/react.development.js"></script> <script crossorigin src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script> <script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script> <script type="text/babel" src="./app.js"></script> </body> </html>

Sheila Anguiano
Full Stack JavaScript Techdegree Graduate 35,239 PointsHi Erika, it would be easier to read if you use markdown, so we can read the code correctly formatted like this:
const Header = (props) => {
return (
<header>
<h1>{ props.title }</h1>
<span className = "stats"> Players: { props.totalPlayers } </span>
</header>
);
}
Instead of this:
const Header = (props) => { return ( <header> <h1>{ props.title }</h1> <span className = "stats">Players: { props.totalPlayers }</span> </header> ); }
Is a best practice
when asking coding questions, and you'll get answers faster
KRIS NIKOLAISEN
54,972 PointsKRIS NIKOLAISEN
54,972 PointsIf you are using a workspace can you post a snapshot? Click the camera icon in the upper right corner, then 'Take Snapshot', then post the link created here.