Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial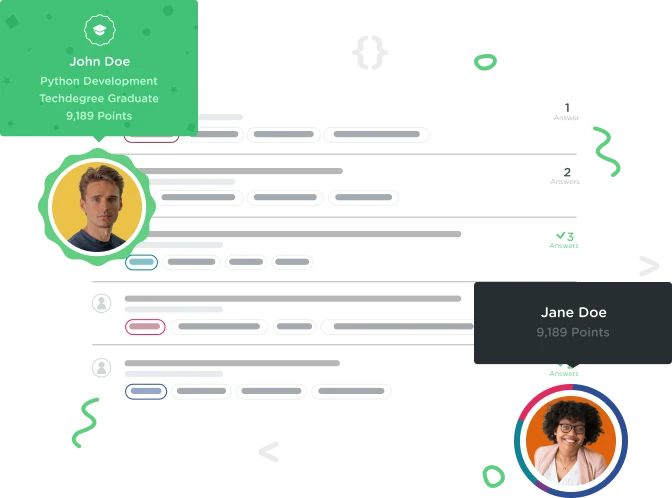
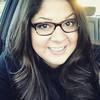
Nicole Archambault
27,853 PointsWhy aren't the $pronoun and $verb variables being set/picked up in this function?
I made a modified version of one of the PHP lessons to challenge myself, and seem to have made everything work except for assigning pronouns and verbs based on whether the name is mine (Nicole = she, is) or any other (they, are).
For some reason, it's totally omitting $pronoun and $verb. I am very new to this (just started yesterday), so could someone clue me in as to what I'm doing wrong, and what I could be doing more efficiently?
Thanks!
<?php
function get_info($name, $title = Null){
$title_announce = " $pronoun $verb with us as a $title. Welcome, $name!";
//Checks if name entered was Nicole
if($name == 'Nicole'){
$pronoun = 'she';
$verb = 'is';
echo "$name has arrived!";
//Checks to see if the title was included in input, to add title announce.
if( $title != Null ){
echo $title_announce;
} else { }
//If name entered was not Nicole
} else {
$pronoun = 'they';
$verb = 'are';
echo "$name has arrived from far away!";
//Checks to see if the title was included in input, to add title announce.
if( $title != Null ) {
echo $title_announce;
} else { }
//Do nothing
}
}
get_info('Nicole', 'web application developer');
1 Answer
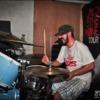
Mike Costa
Courses Plus Student 26,362 PointsHey Nicole,
I re-wrote this for you to minimize your code and more efficiently.
<?php
function get_info($name, $title = null){
$pronoun = 'they';
$verb = 'are';
$arrived_message = " has arrived from far away!";
if($name == 'Nicole'){
$pronoun = 'she';
$verb = 'is';
$arrived_message = " has arrived!";
}
echo $name . $arrived_message;
if( !is_null($title) ){
echo "$pronoun $verb with us as a $title. Welcome, $name!";
}
}
get_info('Nicole', 'web application developer');
When you first enter the function, I'm setting the proverb, verb and arrived message variables to something if the name does not equal Nicole. This way you won't need an else statement. If the name IS Nicole, then you can override those 3 variables. However, if this was a big application and there was more variables, this would not be the best route to go, but for simplicity sake and teaching, I decided this would be a good way to understand the way variables get set.
So once the function runs, those 3 variables
$pronoun = 'they';
$verb = 'are';
$arrived_message = " has arrived from far away!";
get set. So if $name does not equal Nicole, it will never look inside of that if statement to re-assign them. Next I echoed out the concatenation of the $name var with the $arrived_message var. If you are unfamiliar with concatenation, its basically the process of "sticking" 2 (or more) string types together with a period. For instance,
echo "I had a " . " lovely time"; // I had a lovely time
$name = 'Mike';
$message = "Hello, your name is";
echo $message . " ------ " . $name; // Hello, your name is ------ Mike
Then I checked if $title was not null using a native php function is_null() with the NOT operator (or !). The NOT operator (for lack of a better way to describe this) will negate or make opposite of what you are trying to check. For instance,
$title = null;
is_null($title) // this equal to true
!is_null($title) // this will equal to false (which will never reach inside the if statement above in the code).
$name = 'Nicole';
is_null($name) // this will be false because $name has a value
!is_null($name) // this is true
I hope this helps!! :)
Nicole Archambault
27,853 PointsNicole Archambault
27,853 PointsI imagine that this has to do with order of operations, and the fact that $pronoun and $verb need to be defined beforehand, so I'd need to reorder everything...