Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial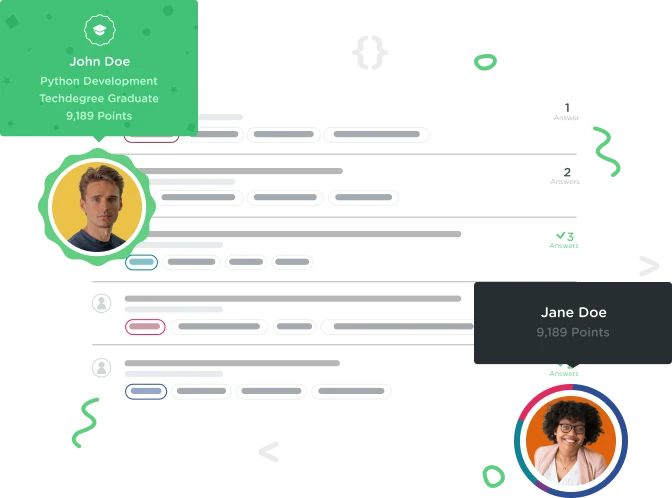

Daniel Zmak
4,476 PointsWhy ask to try a different number if player is out of tries?
It didn't make sense to me that after a player has exhausted their number of guesses the game would still print out "Try a higher/lower number" before also saying "Sorry, you didn't get it..."
In order to eliminate that I added an if statement in the same indentation as the first while loop, but is there an easier way to do this?
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 2:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("Sorry, that is not a number!")
else:
if guess == secret_num:
print("You got it! The secret numer is " + str(secret_num))
break
if guess > 10:
print("""GAME RULES:
Please choose a number from 1 through 10.""")
if guess > secret_num:
print("Try a lower number.")
else:
print("Try a higher number.")
guesses.append(guess)
if len(guesses) == 2:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("Sorry, that is not a number!")
else:
if guess == secret_num:
print("You got it! The secret number is " + str(secret_num))
if guess != secret_num:
print("Sorry, you didn't get it. The secret number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()
[MOD: added ```python markdown formatting -cf]
4 Answers
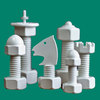
Steven Parker
229,783 PointsIt looks like a chunk of code got replicated. There shouldn't be two places in the loop asking the same question. Also, be aware that when you add a line with the same indentation as the while, that makes the previous line the end of the loop and your new line comes after the loop.
Here's a revised version with the replicated part removed (and a few minor fixups):
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 2:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("Sorry, that is not a number!")
else:
if guess == secret_num:
print("You got it! The secret number is " + str(secret_num))
break
if guess > 10:
print("""GAME RULES:
Please choose a number from 1 through 10.""")
continue
if guess > secret_num:
print("Try a lower number.")
else:
print("Try a higher number.")
guesses.append(guess)
if guess != secret_num:
print("Sorry, you didn't get it. The secret number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()

Daniel Zmak
4,476 PointsThanks for your answer Steven. However, this still doesn't solve my problem. On the last try, the response should skip asking you to try a lower/higher number, and instead just tell you that you didn't get it right.

Kiko Augusto
2,764 PointsJust append the guess into guesses right after the user input and put the if len(guesses) == 3 inside the guess comparison.
while len(guesses) < 3:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("Sorry, that is not a number!")
else:
guesses.append(guess)
if guess == secret_num:
print("You got it! The secret numer is " + str(secret_num))
break
if guess > 10:
print("""GAME RULES:
Please choose a number from 1 through 10.""")
if guess > secret_num:
if len(guesses) == 3:
continue
else:
print("Try a lower number.")
elif guess < secret_num:
if len(guesses) == 3:
continue
else:
print("Try a higher number.")
else:
print("Sorry, you didn't get it. The secret number was {}.".format(secret_num))
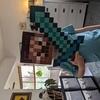
Gabbie Metheny
33,778 PointsMy solution is similar to Kiko's, but I created a tries variable so that if you wanted to change the number of tries the player had, you would only have to change the number once in your code.
I append guess to guesses after testing to see if guess == secret_num; the lower or higher suggestions will only print out if the player has any remaining tries. If not, the else block will run, telling the player they did not guess correctly. I tried pretty hard to break the program after this addition, and I couldn't, so I think it's solid.
import random
def game():
# generate a random number between 1 and 10
secret_num = random.randint(1, 10)
guesses = []
tries = 5
while len(guesses) < tries:
guess = input("Guess a number between 1 and 10: ")
try:
# get a number guess from the player
guess = int(guess)
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
# print hit/miss
print("You got it! My number was {}.".format(secret_num))
break
guesses.append(guess)
if len(guesses) < tries:
if guess < secret_num:
print("My number is higher than {}.".format(guess))
elif guess > secret_num:
print("My number is lower than {}.".format(guess))
else:
print("You didn't guess it! My number was {}.".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()