Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial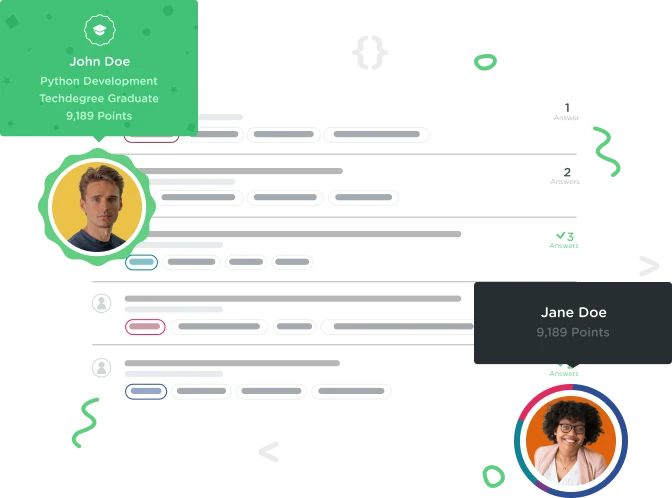

self learner
1,556 PointsWhy at 7:50 didn't remove the whole list items?
fruits = ['apple', 'banana', 'mango', 'orange', 'pears']
for item in fruits:
fruits.remove(item)
print(fruits)
Output:
['banana', 'orange']
4 Answers

diogorferreira
19,363 PointsCraig explains it at the end of the video a little better, but basically it's skipping every second item in the array because every time you delete an item it shifts to the left by one and the array index moves to the right by 1 as it's starting a new loop.

Mirza Gogic
4,342 PointsUse pythontutor.com to visualize the execution of the code.
The way I understand it the lists are mutable, and they change. This is what happens:
fruits = ['apple', 'banana', 'mango', 'orange', 'pears']
for item in fruits:
fruits.remove(item)
print(fruits)
Once the first round of looping is done at fruits.remove, "apple" is gone and "banana" moves to it's spot. So "apple" was 0, and once it is gone, "banana" is 0. The looping continues, and the code goes on to check spot number 1. And there we have "mango" as the elements went to the left ("banana" now being on position 0, and "mango" on 1). That is why the next place the code executes is position 1, and "mango" is removed. Now "orange" takes position 1, and "pears" position 2. The code won't check the position 1, but it goes on and removes position 2. which is now "pears".
Does it make any sense?
This is just my opinion on how we got here, hope someone smarter can check and confirm if it is true.

Oscar Coello
2,181 PointsThanks for posting that pythontutor website! I am a visual learner and it helps tremendously!

Ratan Singh
1,440 PointsYou are absolutely right!

diogorferreira
19,363 PointsAt index 0 of the loop it removes apple
, but then when it goes for another iteration it would be at index 1 (second loop) and the list would be like this:
['banana', 'mango', 'orange', 'pears']
Meaning instead of removing banana, it removes mango because it's at index 1.
This then repeats for orange and that's why you are left with orange
and mango
.
You can fix this by looping through a copy of the array like Craig shows. This is because the array stays the same, it will never change

self learner
1,556 PointsCan you pls elaborate little more?
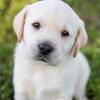
Chotipat Metreethummaporn
1,129 PointsAccording to diogorferreira, so if we use fruit_list.remove("mango"), everything in the copy list will not change? I mean its index is still the same? Am I right?
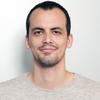
Nemanja Savkic
17,418 PointsEach time it runs through the list it increments the index by 1 (to get to each element) but also removing one item from the list
List before running the loop: 0 1 2 3 4 apple banana mango orange pears
apple is removed in the first round, the loop counter is now 1, which is now moved to mango 0 1 2 3 banana mango oranges pears
it removes mango and the counter is now 2 - pears 0 1 2 banana oranges pears
Eric Slater
6,990 PointsEric Slater
6,990 PointsThank you! I didn't quite get that. I understand now why it is only removing every other item when looping through the list, but I still don't understand why it works when looping through the copy. Wouldn't the copy exhibit the same issues when removing items while looping?