Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial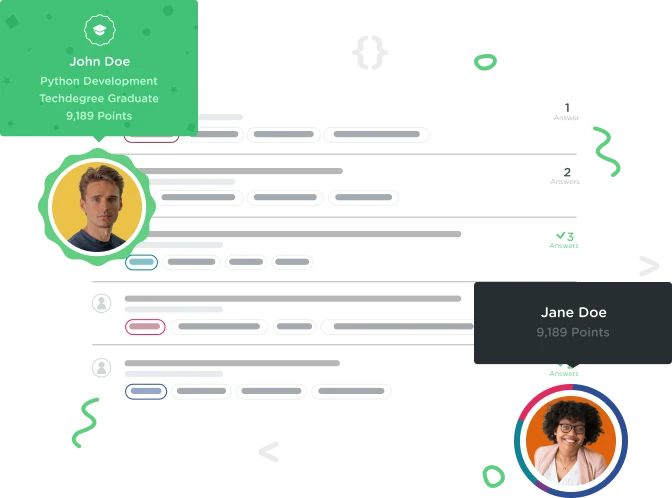
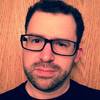
Chris Shaffer
12,030 PointsWhy call console.log on a function when it's already logged to the console? Seems redundant, same effect.
In the Javascript Foundations, "Return Values" video you show a number of variations on a simple function.
By calling that function, the output is logged to the console. Let me give an example:
function someName (name, greeting) { console.log(name + " something " + greeting); };
someName("blah", "hello")
As part of this function, it's ALREADY logged to the console, in fact, you could argue it's function IS to log to the console, yet later in the video you say we're going to wrap the function call in a console.log(), but don't explain WHY.
The result is the EXACT same whether or not you call the function on it's own or not; the output is logged to the console.
You added a RETURN statement to the function, which is then logged now that you called console.log on the function call, in this case, return name.length.
I'm unclear as to the purpose of using console.log again other than the name.length and I'm confused why it isn't redundant; why doesn't it print out the already console-logged stuff twice?
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsI think you might be slightly misunderstanding how function calls work.
Let's say you have a function like this:
function firstFunction() {
console.log("Output from firstFunction");
}
Calling firstFunction()
will output the string that has been passed to console.log
, but the function itself will not return a value as there is no return statement. Such functions return undefined
.
If you were to try this function in Chrome Web Developer Tools, the output would look like this:
> firstFunction();
Output from firstFunction
<- undefined
Note the little arrow (<-
) in front of undefined
. That's Chrome's way of telling you that it's displaying the return value of the function you called, but only for debugging purposes. The (nonexistent) return value of that function hasn't been explicitly logged to the console and some other JavaScript environment wouldn't print it out at all. That's why it's prefixed with the arrow, to show that it's a return value printout for easier debugging.
Now, let's expand on that function:
function secondFunction() {
console.log("Output from secondFunction");
return "Second function return value";
}
This function logs something to the console, just like firstFunction
but it also adds a return value. So, calling this results in:
> secondFunction();
Output from secondFunction
<- "Second function return value"
Now, undefined
has been replaced with the functions actual return value. The arrow is there again, to signify that it's a return value, but also take note of the fact that "Second function return value"
is wrapped in quotes unlike the output. That signifies that the return value is a string.
Now, if you were wrap the call to the secondFunction
function in a console.log
statement, this is what would happen:
> console.log(secondFunction());
Output from secondFunction
Second function return value
<- undefined
The first line is your call to console.log
. You are passing the call of secondFunction
as a parameter to console.log (it's important to note that you're not passing the function itself, just a call to the function — that means the actual parameter will be the return value of secondFunction
).
When secondFunction
is called, it prints out Output from secondFunction
. It's something that happens inside that function and is restricted to that function. That string doesn't get passed anywhere. This is what is called a function side effect.
Functions in programming generally behave like mathematical functions. You have inputs and outputs. For a set of inputs you'll get an output. The inputs are the function parameters, and the output is the return value. But sometimes, we're not interested in what the function returns, we just want the function to do something on its own (change some other value, print something out, store data on the disk, etc). This is what is called a function side effect.
So, the appearance of Output from secondFunction
is a side effect of calling secondFunction
but that string doesn't reach the console.log from the first line.
The second line of output (Second function return value
) is the return value of secondFunction
being printed out by the console.log
call wrapped around the call to secondFunction
. When you call a function and pass it to another function as a parameter, the other function will be working with the return value, not the function itself.
So, Second function return value
appears in the console because it was explicitly printed out by the console.log
and not because Chrome Web Developer Tools are trying to be helpful.
However, the Developer Tools are to blame for the last line of the output. From the arrow, you can deduce that it's a return value. That value is being returned (or rather nothing is being returned) from console.log
.
When talking about functions that have side effects, console.log
is completely about side effects — it prints to the console, but it doesn't return anything. That's why the return value is undefined
.
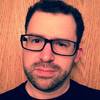
Chris Shaffer
12,030 PointsTHANK YOU! This is by far the best explanation of a function I've ever had and I totally understand now!
You just blew my mind, man!
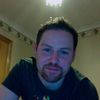
Jason Mc Dermott
11,496 Pointsthat was an awesome answer, thank you so much