Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial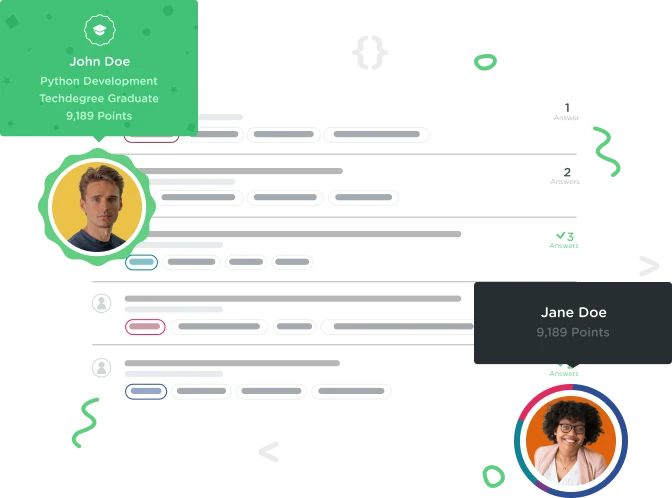
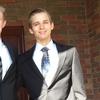
Gavin Broekema
Full Stack JavaScript Techdegree Student 22,443 PointsWhy call enableSubmitEvent()?
Original app.js code:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
////Hide hints
//$("form span").hide();
function isPasswordValid () {
return $password.val().length > 8;
}
function arePasswordsMatching () {
return $password.val() === $confirmPassword.val();
}
function canSubmit () {
return isPasswordValid() && arePasswordsMatching();
}
function passwordEvent () {
//Find out if the password is valid
if (isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent () {
//Find out if password and confirmation match
if (arePasswordsMatching()) {
//Hide hint if matched
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
enableSubmitEvent () {
$("#submit").prop("disable", !canSubmit());
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
Why call enableSubmitEvent() when you could just add the disabled prop in HTML?
<input type="submit" value="SUBMIT" id="submit" disabled>
Same thing applies with adding:
$("form span").hide();
vs. changing the CSS to:
span {
display: none;
}
^^^Especially considering that when this part of the page is run with jQuery, the "hidden" hint spans flash before the window as the page loads. When I ran it with CSS the the hint spans didn't show up until the input elements gained focus.
2 Answers

Christopher Janzen
9,417 PointsI could be wrong but I'd also venture to guess that if you put the disabled property on the submit button in html and somebody had JS disabled then they wouldn't be able to submit the form because the code would never run and therefore wouldn't remove the disabled property. I'd treat this whole scenario as a progressive enhancement rather then a genuine way of testing for form validity. You'd still want to use some type of backend code to check to see if the form is properly filled out. The JS is just a way to help the user through the form.

jennyhan
36,121 PointsThat's a default setting. The button is set to be disabled by default so that you can't submit even you entered nothing.
nathanmendes
6,929 Pointsnathanmendes
6,929 PointsI think it's because calling canSubmit() gives you a true or false value depending on if the passwords match or not, but doesn't actually change the property value inside the submit button. By calling the enableSubmitEvent() function, you actually change the property value of "disabled" in the submit button.