Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial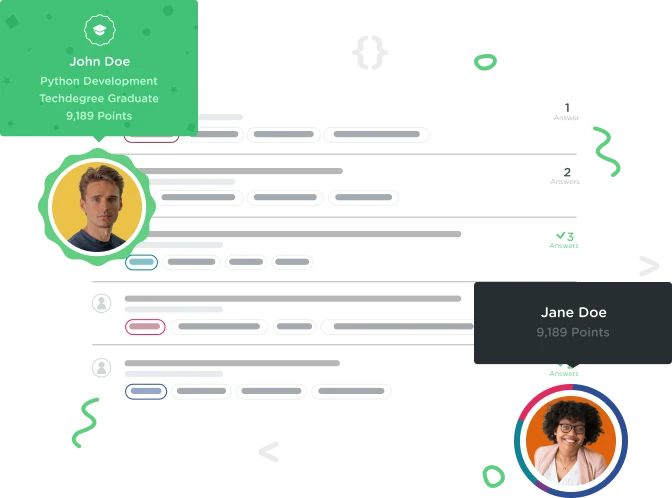
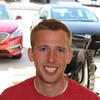
steven swensen
7,926 PointsWhy can I not use an arrow function in an object?
why does this work.
var dice = {
sides: 6,
roll: function () {
let random = Math.floor(Math.random() * this.sides) + 1;
return random;
}
}
var show = document.querySelector('#dis');
var button = document.querySelector('#go')
.addEventListener('click', () => {
var RN = (dice.roll())
show.innerHTML = RN;
});
but this doesn't
var dice = {
sides: 6,
roll: () => {
let random = Math.floor(Math.random() * this.sides) + 1;
return random;
}
}
var show = document.querySelector('#dis');
var button = document.querySelector('#go')
.addEventListener('click', () => {
var RN = (dice.roll();
show.innerHTML = RN;
});
to be more specific the roll function on line 3
3 Answers

Joseph Wasden
20,406 PointsRegular functions declared using the function
keyword have their own this
. After some digging, I learned that arrow functions do not have their own this
. For this reason, they don't make good methods on objects.
If you use a this
keyword inside an arrow function, it references the context of where the call was made. In this case, that is the global scope. This means that your this.sides
reference isn't pointing where you intend when the roll()
function is called.
If you add var sides = 6
to the global scope, then the code with the arrow function resolves as expected.
For this reason (among others), arrow functions aren't recommended to be used as methods on objects, per MDN.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/Arrow_functions
Here's a code example to demonstrate.
var sides = 6; //declared in global scope
window.onload = () => {
var dice = {
//sides: 6, commented out for demo purposes
roll: () => {
let random = Math.floor(Math.random() * this.sides) + 1;
return random;
}
}
var show = document.querySelector('#dis');
var button = document.querySelector('#go')
.addEventListener('click', () => {
var RN = dice.roll(); // removed stray open parenthesis, hat-tip to Steven Parker
show.innerHTML = RN;
});
}
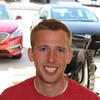
steven swensen
7,926 PointsThat clears things up big league. Thanks Joseph.

Joseph Wasden
20,406 PointsThat arrow functions don't have their own this
was news to me. Thanks for asking a great question.
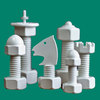
Steven Parker
231,198 PointsThere are other differences also. Check out the Arrow Functions documentation page.
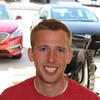
steven swensen
7,926 PointsAwesome thanks Guys and good to hear from you Steven.