Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial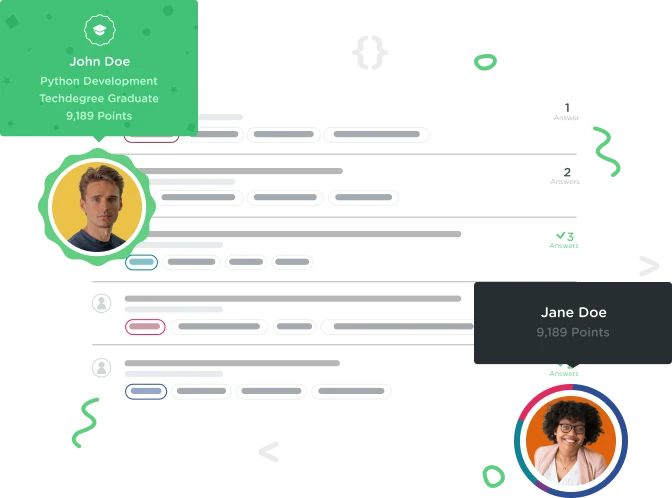

Auceanne Richer
513 PointsWhy can't I add the index to the end of the print for-in loop?
I'm not sure why the list isn't printing the for-in loop, where I added the index at the end of my print for-in loop. Why doesn't this work?
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
print("Continents:")
for continent in continents:
print("* " + continents[0, 3, 5, 6])
1 Answer
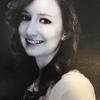
Megan Amendola
Treehouse TeacherHi Auceanne Richer ! When you pass something into a list (like my_list[something]
), it can only be an integer or a slice. What you have passed into the list right now is a tuple (which you will learn about later if you're on the Python track). This will cause its own error.
Let me break down what happens in your loop. If I print continents, I am printing out the whole list equal to the number of items in the list.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
print(continents)
# console
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
>>> [ 'Asia', 'South America', 'North America', 'Africa', 'Europe', 'Antarctica', 'Australia',]
This is because a loop repeats equal to the number of items in the list (or other iterable) you give it. Here the iterable is the continents list which has 7 items in it, so the loop repeats 7 times and does whatever is inside of the loop 7 times.
What the challenge wants you to do is print out a bulleted list of all of the continents using a loop. If we instead print out continent
instead of continents
, this is what you'll see.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
print(continent)
# console
>>> Asia
>>> South America
>>> North America
>>> Africa
>>> Europe
>>> Antartica
>>> Australia
Now, you get each continent printed out to the console because continent
changes each loop to be the next item in the loop. Then you just need to add '* ' +
to get them as bullets.
The second task asks you to only print out continents that start with 'A'. In your loop, continent is a string because you have accessed an individual string from the list. With strings, you can use index notation to determine what the first letter is, like this:
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
print('* ' + continent[0])
# console
>>> * A
>>> * S
>>> * N
>>> * A
>>> * E
>>> * A
>>> * A
Now all you need to do is print out the continent only if the first letter is 'A'.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
# if first letter equals 'A'
print('* ' + continent)
Hopefully, this helps. Let me know if you're still stuck