Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial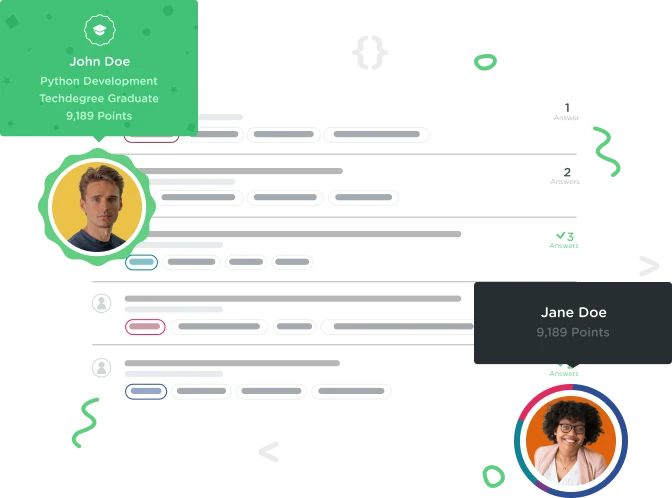
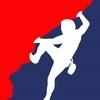
Greg King
4,073 PointsWhy can't I create the 'result' variable in the same line as the typecast?
Why does this following block not work? (Specifically the line: String result = (String) obj;)
if(obj instanceof String) {
String result = (String) obj;
}
else if(obj instanceof BlogPost) {
BlogPost blog = (BlogPost) obj;
result = blog.getTitle();
}
It doesn't let me declare 'String result' and cast it in the same line. I have to declare the string variable first and then typecast like this:
String result = "";
if(obj instanceof String) {
result = (String) obj;
}
else if(obj instanceof BlogPost) {
BlogPost blog = (BlogPost) obj;
result = blog.getTitle();
}
I want to know why the first method does not work. Afterall, it works for:
BlogPost blog = (BlogPost) obj;
so why would it not be the same for:
String result = (String) obj;
1 Answer
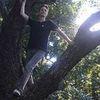
gyorgyandorka
13,811 PointsThe problem is not with typecasting, but with scope: technically you can declare a variable inside the if
-block and initialize it on the same line, but it will only be visible inside the block - the else if
branch will not see it and cannot use it. That's why you should first declare result
above.
In case of blog
, you can declare and initialize it inside the block, since you only intend to use it on the next line, still inside the else if
block, in order to get the return value from getTitle()
and assign it to result
- which is the variable we'd like to return in the end (and, again, must be declared outside therefore)