Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial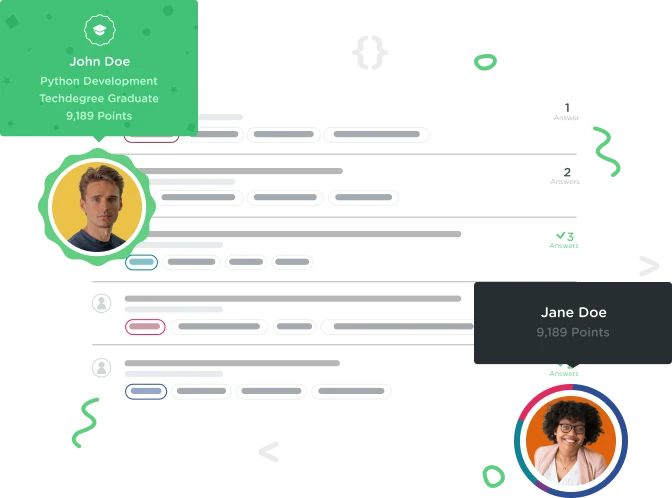
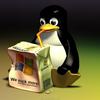
Dennis Saadeddin
1,303 PointsWhy can't I declare this object outside the 'if' statement?
Hey guys! So in the following code I can't declare the 'blogpost' object before the second 'if' statement.
import com.example.BlogPost;
public class TypeCastChecker {
public static String getTitleFromObject(Object obj) {
if(obj instanceof String)
return (String) obj;
if(obj instanceof BlogPost) {
BlogPost blogpost = (BlogPost) obj;
return blogpost.getTitle();
}
return "";
}
}
I know that it is not recommended to declare 'blogpost' inside the if-statement. Is that true? Looking forward for your answers! :D
3 Answers
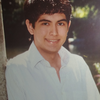
Jon Kussmann
Courses Plus Student 7,254 PointsHi Dennis,
I'm not sure I understand your question. If you have:
import com.example.BlogPost;
public class TypeCastChecker {
public static String getTitleFromObject(Object obj) {
BlogPost blogpost;
if(obj instanceof String)
return (String) obj;
if(obj instanceof BlogPost) {
blogpost = (BlogPost) obj;
return blogpost.getTitle();
}
return "";
}
}
It should work. Are you getting some sort of error?
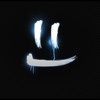
Grigorij Schleifer
10,365 PointsHi Dennis,
if you declare the blogpost inside the if statement the blogpost will not be visible outside the if-block.
I am not sure about it, but it seems logic to me.
And if you cast the obj to blogpost, the compiler knows that obj is a blogpost ... because you are casting from the Object class to the BlogPost class.
Tell us your progress
Grigorij

Rey Adronico Baguio
13,672 PointsI'm more confused if the blogpost object was declared outside.
My additional question if you declare the new blogpost object outside is: can we leave new objects of a non-native classes, such as the BlogPost class, undeclared such as what Jon suggested or should we add a "new BlogPost" leaving the inputs blank?
For me, I instantiated the new BlogPost inside the if statement then declared it as a typecasted "obj". For the purpose of the exercise, it's fine. But in future projects, do know that variables instantiated (and/or declared) INSIDE the if statement are accessible ONLY in that if statement (not sure if you can access it in subsequent else-if or else statements).
Dennis Saadeddin
1,303 PointsDennis Saadeddin
1,303 PointsHi Jon,
So I tried to use your approach in the challenge, but the compiler returned an error. I'm sorry for the ambiguity of my question. My actual question is: Is it better to declare the 'blogpost' object outside the if statement or inside? Does it really matter where it is declared? Also, when I cast it
blogpost = (BlogPost) obj;
'blogpost' will from now on be an 'obj' object? I'm getting a little confused. :D
Jon Kussmann
Courses Plus Student 7,254 PointsJon Kussmann
Courses Plus Student 7,254 PointsWhat does the error say?
Depending on the situation, it might be better to declare a variable outside an if-statement, in another it might be better to declare it inside. If you are only using the variable inside one part of the if-statement, just declare it there. If you have to use the variable for other operations, you might need to declare it outside. In this case you should just declare it within the if-statement.
blogpost = (BlogPost) obj;
Assuming you've declared your blogpost variable elsewhere:
Before this line of code we know 'obj' is from the Object class. We are casting the reference of 'obj' into a BlogPost. We are then assigning the variable 'blogpost' to the reference of 'obj' as a BlogPost.
Your question: 'blogpost' will from now be an 'obj' object?
blogpost will be an object from the BlogPost class. Since BlogPost inherets from Object, blogpost will also be an Object. 'obj' is only an object from the Object class.
It's a little difficult to understand. Fido is an object of the Dog class. Fido is also an object of the Animal class (since Dogs are Animals). Charlie is an object of the Animal class, but you cannot say that Charlie is a Dog. You would have to use the "instanceof" operation and then cast Charlie to a Dog.
referenceToNewDog = (Dog) Charlie;
Let me know if I can clarify further.
Quinton Rivera
5,177 PointsQuinton Rivera
5,177 PointsCan you please tell me the code for only the first section which ask: The method getTitleFromObject will be called and passed a String and/or a com.example.BlogPost. For this first task, return the object obj type casted as a String if it is in fact a String.
My code is not working for the first challenge: public static String getTitleFromObject(Object obj) {
}
Quinton Rivera
5,177 PointsQuinton Rivera
5,177 Pointsdoes the return "", cant explain it what does the last line of code do, does it declare it a null return until until the obj is casted to either a blog post or string?