Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial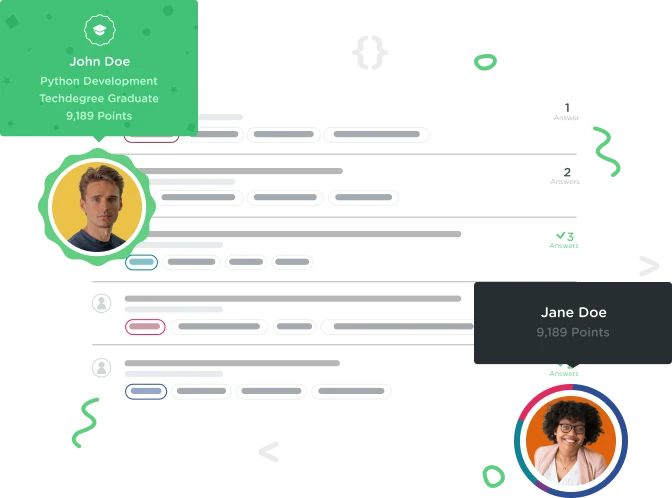

Logan Hutchins
6,680 Pointswhy cant i delete the list from the list?
this is what i used, it looks correct?
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.pop(3)
messy_list.insert(0, 1)
messy_list.remove(["a", False])
del messy_list(5)
3 Answers
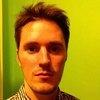
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 Pointsmessy_list.pop(3)
messy_list.insert(0, 1)
This passed the first part of the challenge, but it’s not ideal. In this case, you happen to know that the value you’re removing is 1, so you’ve hard coded that in. But you want code that is flexible and can apply to lots of situations, so that you could remove an item a certain index and insert it at another index without knowing its value. You could do it like this:
item = messy_list.pop(3)
messy_list.insert(0, item)
or all in one line:
messy_list.insert(0, messy_list.pop(3))
For your next line:
messy_list.remove(["a", False])
The remove method takes one argument, the value you want to remove. The value you’ve passed in is this array [“a”, False]
. Those two items in the array exist individually in messy_list, but the array as an item doesn’t, and that’s what it’s checking. You’re going to have to pass those values in individually into the remove method.
And the last line:
del messy_list(5)
Since messy_list
isn’t a function, it’s a list, you refer specific items in it with square brackets [] not parentheses. Also keep in mind that after you’ve removed/deleted items from this list, the index of the item you’re looking for may have moved from its original state and will have a different index. What's handy about the remove()
method is that you don't need to know the index, just pass in the value.

Logan Hutchins
6,680 Pointsi tried your suggestions, it all looked really good,but they dont seem to work, i even .removed everything individually but it still wont pass
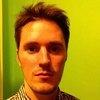
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 PointsThis passes Challenge Task 2 of 2 for me:
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0, messy_list.pop(3))
messy_list.remove("a")
messy_list.remove(False)
messy_list.remove([1, 2, 3])
But if you're starting back at Task 1 of 2 take out the last 3 lines. It needs the list in the earlier state for that step to pass.

Logan Hutchins
6,680 Pointsthat worked. i had something very similar except i removed the numbers seperately. probably a typo on my part "lost some syntax master obi wan has, how embarrassing...."