Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial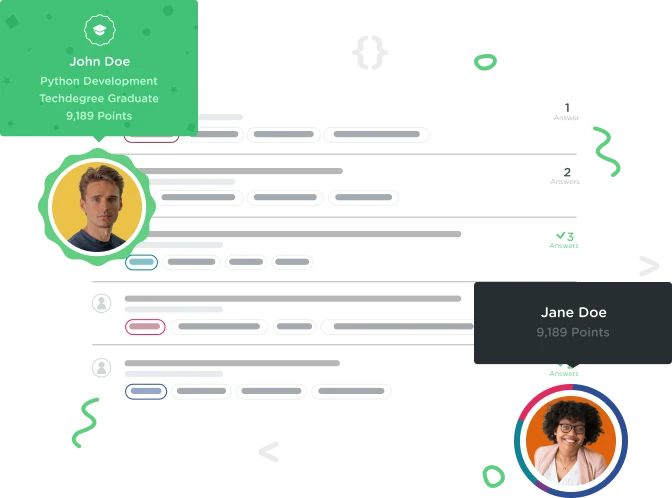

Johnny Harrison
4,331 PointsWhy can't I get the code to run with only one slash '/'
I Googled // vs / and found that (in Python 3) // is used for integer division, and / is used for float division.
When I try to run this code:
def yell(text):
praise = text.upper()
number_of_characters = len(text)
result = text + "!" * ( number_of_characters / 2 )
print(result)
I get a TypeError: can't multiply sequence by non-int of type 'float'
Fair enough... I'll just change both numbers from ints to floats like this:
def yell(text):
praise = text.upper()
number_of_characters = len(text)
result = text + "!" * ( float(number_of_characters) / 2.0 )
print(result)
When I run that, I get the same error and I can't see why that would be.
Even if I open the python shell and type '5/5' or something, it still converts them to floats and returns 1.0, so I shouldn't even need to go out of my way to change the variables. It's gotta be something else going on, I'm just not sure what.
3 Answers
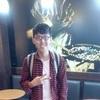
Samuel Zhen
33,571 PointsAccording to the error message TypeError: can't multiply sequence by non-int of type 'float', I'm pretty sure python doesn't allow us to print a string multiple times based on a float number.
If we think about it, It kinda make sense. Imagine the number_of_characters is 9. What is the result of the division?
result = text + "!" * ( number_of_characters / 2 )
It will return 4.5, a float number. And we try to print "!" 4.5 of times? Like, how many exactly is that?
So we use // to make sure we multiple the string with integer number.
I hope this will help you.

Johnny Harrison
4,331 PointsI found the solution soon after posting this thanks to this similar thread.
The part that was causing it to error was the "!" being added to it. If you test by opening the python shell and typing something like
"!" + 1.0
you'll see that it won't work because strings can't be added to floats. But, strings can be added to ints. For example:
"!" + 1
will return
'!'
as expected.

KRIS NIKOLAISEN
54,971 PointsIf strings can be added to ints why does
print("!" + 1)
return a type error