Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial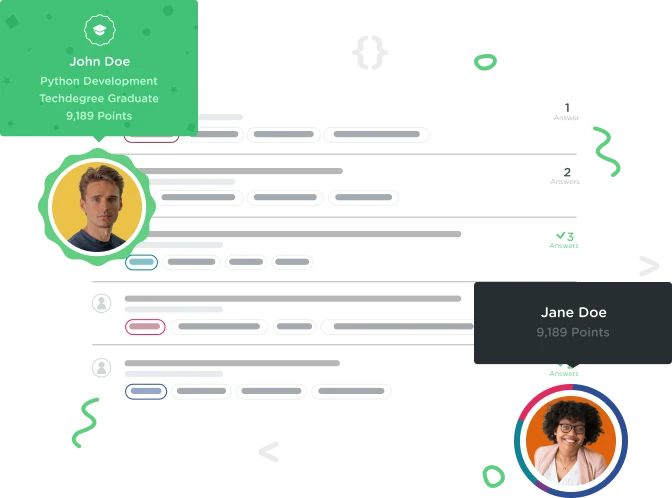
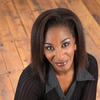
Samantha Atkinson
Front End Web Development Techdegree Graduate 40,307 PointsWhy can't I give setInterval function a delay time of 0/zero.
Is it really bad to give the setInterval function a delay of 0 seconds? My thinking is our getTime() function is getting the current time right? So if the delay is set to 0 then getTime will start with no delays and continue displaying the seconds.
const clockSection = document.getElementById("clock");
function getTime() {
function pad(number) {
if (number < 10) {
return "0" + number;
} else {
return number;
}
}
const now = new Date();
const hh = pad(now.getHours());
const mm = pad(now.getMinutes());
const ss = pad(now.getSeconds());
return `${hh}:${mm}:${ss}`;
}
setInterval( () => clockSection.textContent = getTime(), 0);
Ā
Hi @Steven Parker I saw in one of your answers that you said when an arrow function contains more than just an expression (as this one has an assignment), you should place parentheses around the function body.
I have done the same mistake because I thought the rule was if there is only 1 statement in an arrow function you can remove the curly brackets to make it more concise?
2 Answers
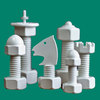
Steven Parker
231,275 PointsIt's not parentheses, but braces ("curly brackets") that you would use when you have more than just an expression in an arrow function. Please post a link if you saw something where I said otherwise. In that case, you can have any number of complete statements inside the braces, and you can use a return
statement to pass back a value. Since you have a complete statement (assignment), you should have braces around it.
Without the braces, you can only have an expression (not a statement), and the value it generates will be returned automatically.
As a completely separate issue, you can certainly have 0 as a delay, but you probably won't want to. Without a delay, the callback will be invoked over and over as fast as possible, consuming massive CPU resources. If your intent is to have something happen once per second, the delay should be 1000. If you don't want to wait a second for the first update, that's why you'd call it once before starting the interval.
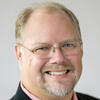
Jason Larson
8,361 PointsIn SetInterval()
, the delay is an optional parameter, so you can set it to 0 or just leave it out entirely.
I'm not sure where you saw the comment from Steven Parker, but that is not correct. The only time you have to put parentheses around the expression of an arrow function is when it is returning an object literal (per the MDN page on Arrow function expressions). I suspect that what was intended in the response was to say that curly braces are required around the function body when there is more than one expression, which is what you said. Note also that when you have multiple statements (and thus require the curly braces) you also have to put the return
statement in, as it is implied with the single expression version without curly braces.
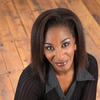
Samantha Atkinson
Front End Web Development Techdegree Graduate 40,307 PointsThank you Jason Larson for your reply. I still don't get why we couldn't or shouldn't use an anonymous function above?
Andrew says not to that it's better to call the tickCloak function first and then use it as a callback function in the setInterval()
.
I searched to see if anyone else had asked the question I just asked in relation to this video. Steven Parker answers are always correct and I saw his answer to a question related to this video mentioning arrow functions. Although now I owe Steven an apology as he was talking about parentheses and not curly brackets š¤¦š¾āāļø, sorry Steven
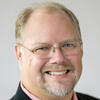
Jason Larson
8,361 PointsIt looks like there is some inherent delay that is being used on setInterval, whether you use it with the named function or as an anonymous function. If you put in your code and then preview several times, you will see the text very briefly when the page first loads. By having the function and calling it directly, you are avoiding that very small delay, and then the setInterval executes. This is really more about calling the function directly before using it as a callback with setInterval.
Samantha Atkinson
Front End Web Development Techdegree Graduate 40,307 PointsSamantha Atkinson
Front End Web Development Techdegree Graduate 40,307 PointsThank you for your reply, Steven Parker. I'm sorry if I misunderstood you in this post about the arrow function. (https://teamtreehouse.com/community/below-is-a-way-to-not-name-a-function-variable-but-is-it-a-best-practice)
Now I get why I can't just give the setInterval method a value of 0. Excellent explanation, thank you.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsAhh, the suggestion I made in that previous answer as a bit obscure, I'll fix it. What the parentheses would do is cause the assignment to be treated as an expression, which does make the short form work but it's not nearly as clear as using the long form (with braces).