Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial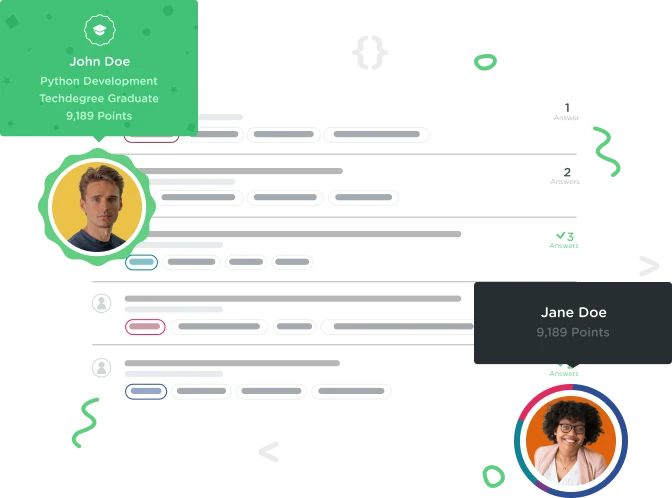
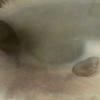
Juan Rodríguez
782 PointsWhy can't I return the counter in this excercise?
I can't seem to return the counter. The excercises asks to increment a counter if there is a tile in the player's hand in a method that is called getTileCount(). So I increment the counter but I CAN'T RETURN IT since the "return" method only returns strings. How can I finish these challenge? I'M STUCKED! In the video we returned a String so there wasn't any problem, in here we must return a count, ergo, an Integer, how can I do it?
public class ScrabblePlayer {
public static String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount()
{
int counter = 0;
for (char tileWeNeedToCount : mHand.toCharArray()){
if (mHand.indexOf(tileWeNeedToCount) >= 0) {
counter += 1;
}
}
return counter;
}
}
3 Answers
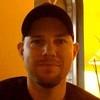
Jeremy Hill
29,567 Pointsin your for loop you need to add a type in there so it knows what type of data to expect:
for(char letter : mHand.toCharArray()) // then in the loop make sure you reference 'letter' or whatever you name it.
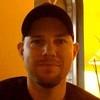
Jeremy Hill
29,567 PointsIt is because you set your method up to return a string:
It should be: public int getTileCount(char tile){ }
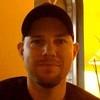
Jeremy Hill
29,567 PointsAlso, you have to give it a char parameter so the counter will know which char to count.
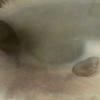
Juan Rodríguez
782 PointsOkay, so I set the method to return an int and I put a parameter for it to accept a char, so that we know which tile we need to count. Like this:
public int getTileCount(char tile) { int counter = 0; for (tile : mHand.toCharArray()) { if (mHand.indexOf(tile) >= 0) { counter += 1; } } return counter; } }
But now it says:
./ScrabblePlayer.java:24: error: bad initializer for for-loop for (tile : mHand.toCharArray()){ ^
So what do I do, why can't I call the char I had set up and use it for the each loop? If I declare it again in the for parameters I will say it's an already-defined variable. So what can I do?
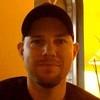
Jeremy Hill
29,567 PointsGood deal! You're welcome :)
Juan Rodríguez
782 PointsJuan Rodríguez
782 PointsThank you a lot! :D That worked just fine.