Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial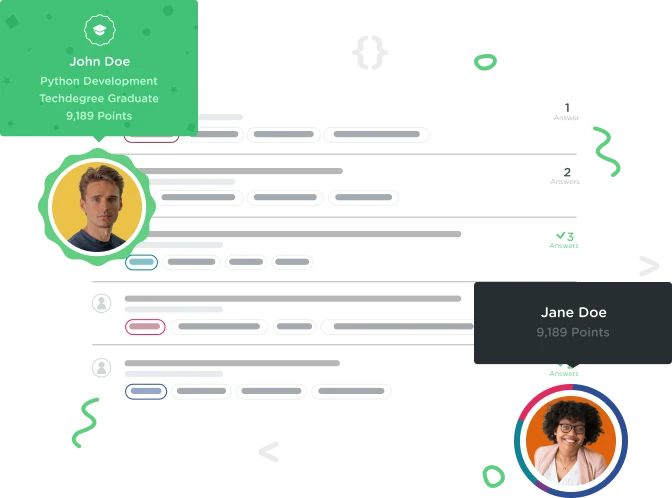

Elliott Tuan
7,790 PointsWhy can't I return the highest price with teacher's method in video?
If I want to return the highest price product, it works.
--
const products = [ { name: 'hard drive', price: 59.99 }, { name: 'lighbulbs', price: 2.59 }, { name: 'paper towels', price: 6.99 }, { name: 'flatscreen monitor', price: 159.99 }, { name: 'cable ties', price: 19.99 }, { name: 'ballpoint pens', price: 4.49 } ];
const highestPrice = products .reduce((highest, product) => { if (highest.price > product.price){ return highest } return product })
console.log(highestPrice) //return { name: 'flatscreen monitor', price: 159.99 }
--
But, if I want to return just a number of price instead an object, it's not work!
--
const products = [ { name: 'hard drive', price: 59.99 }, { name: 'lighbulbs', price: 2.59 }, { name: 'paper towels', price: 6.99 }, { name: 'flatscreen monitor', price: 159.99 }, { name: 'cable ties', price: 19.99 }, { name: 'ballpoint pens', price: 4.49 } ];
const highestPrice = products .reduce((highest, product) => { if (highest.price > product.price){ return highest.price } return product.price })
console.log(highestPrice) //return 4.49
1 Answer
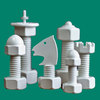
Steven Parker
230,274 PointsThink about what highest represents. In the first example, it is always one of the products objects. But in the second example, after the first iteration it becomes a price value instead. So from then on, the comparison highest.price > product.price
doesn't make sense. The function needs to be modified to make the first argument a price instead of an object. And, this is also a great place to use a ternary expression:
const highestPrice = products.reduce( (highPrice, product) =>
(highPrice > product.price) ? highPrice : product.price,
0); // start with 0 for highPrice
Elliott Tuan
7,790 PointsElliott Tuan
7,790 PointsThank you Parker! I haven't learn about ternary expression, and although I still can't clearly explain the mistake of my thinking in the question upon, I still try to think what you've said "modify the argument to be a price instead of an object", and here's my solution:
I use .map() to make it price value only.
const products = [ { name: 'hard drive', price: 59.99 }, { name: 'lighbulbs', price: 2.59 }, { name: 'paper towels', price: 6.99 }, { name: 'flatscreen monitor', price: 159.99 }, { name: 'cable ties', price: 19.99 }, { name: 'ballpoint pens', price: 4.49 } ];
const highestPrice = products .map(obj => obj.price ) .reduce((highest, price) => { if (highest > price){ return highest } return price })
console.log(highestPrice)
-- Return is right, is my thinking right too?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsSure, that works too. Just for comparison, here's the reduce-only version without the ternary:
And when posting code, always use Markdown formatting to preserve the code's appearance and retain special symbols (as I have done here).
Here's the MDN page for the conditional operator to create ternary expressions.