Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial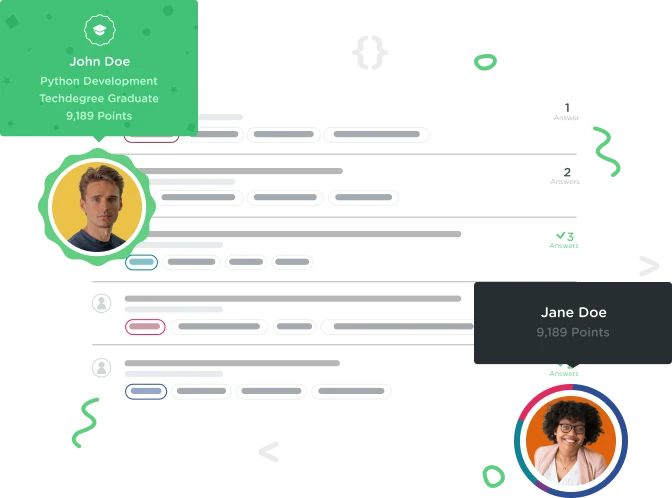
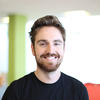
Kristian Woods
23,414 PointsWhy can't I update the textContent of an h3 with my conditional statement?
I have a conditional that checks to see if an item has been added to an array. If that item has been added to the array, I set a previously declared variable "isDouble" from 'false' to 'true'. I then later use another conditional to check if 'isDouble' is true.
If the 'isDouble' is 'false', I want to create a brand new h3 and populate the text with the quantity of that item - if not, I simply want to update the quantity of that item WITHOUT creating a new h3.
The function that is handling all this is called 'addCartItem()' - nearer the end of the code
Can someone please help?
Thank you
(function () {
let body = document.querySelector('body');
let totalBasket = document.querySelector('.totalBasket');
let cartCount = document.querySelector('.cartCount');
let cartItemsDiv = document.querySelector('.cartItems');
function DrinkBluePrint(name, price) {
this.name = name;
this.price = price;
this.quantity = 0;
}
let latte = new DrinkBluePrint('Latte', 5.00);
let flatW = new DrinkBluePrint('Flat White', 3.60);
let cap = new DrinkBluePrint('Cap', 2.75);
let moc = new DrinkBluePrint('Moc', 3.15);
let cortado = new DrinkBluePrint('Cortado', 3.15);
let array = [
latte,
flatW,
cap,
moc,
cortado
];
let cart = [];
let p;
let button;
let isDouble = false;
for (let i = 0; i < array.length; i++) {
p = document.createElement('p');
button = document.createElement('button');
button.textContent = 'Add';
let text = document.createTextNode(array[i].name);
p.appendChild(text);
body.appendChild(p);
body.appendChild(button);
button.addEventListener('click', (e) => {
if (cart.indexOf(array[i]) !== -1) {
isDouble = true;
}
cart.push(array[i]);
displayTotal();
addCartItem();
});
function displayTotal() {
let total = 0;
for (let i = 0; i < cart.length; i++) {
total += cart[i].price;
}
totalBasket.textContent = '£ ' + total.toFixed(2);
cartCount.textContent = `You have ${cart.length} items in your cart`;
if (total >= 10) {
let discountedPrice = (total - 3);
totalBasket.textContent = '£ ' + discountedPrice.toFixed(2);
}
}
// code that needs fixed below
function addCartItem() {
// add one to quantity
addOne();
// create h3 and append text node
let h3 = document.createElement('h3');
let h3Text = document.createTextNode(array[i].name + " " + array[i].price.toFixed(2) + " x " + array[i].quantity);
h3.appendChild(h3Text);
// check to see if item has already been added to cart
if (!isDouble) {
// if item hasn't been added before, append the h3 to the div
cartItemsDiv.appendChild(h3);
} else {
// if item has already been added, then update the text of the existing h3
h3.textContent = array[i].name + " " + array[i].price.toFixed(2) + " x " + array[i].quantity;
}
console.log(h3.textContent);
}
function addOne() {
let itemQuantity = array[i].quantity += 1;
}
};
})();
1 Answer
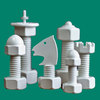
Steven Parker
231,269 PointsThe "h3" that you are updating in the "else" is the same new one you constructed for adding if it was not a duplicate. But since it is a duplicate, it never becomes part of the document.
To update an existing one you need to select it from the document first. That will be easier to do if you mark the ones you create with an ID based on their index number:
h3.appendChild(h3Text);
h3.id= "item" + i; // <-- MARK THE NEW ITEM TO MAKE IT EASY TO FIND
// check to see if item has already been added to cart
if (!isDouble) {
// if item hasn't been added before, append the h3 to the div
cartItemsDiv.appendChild(h3);
} else {
// if item has already been added, then update the text of the existing h3
let h3 = document.getElementById("item" + i); // <-- FIRST SELECT THE EXISTING ONE
h3.textContent = array[i].name + " " + array[i].price.toFixed(2) + " x " + array[i].quantity;
}
Kristian Woods
23,414 PointsKristian Woods
23,414 PointsIs this what you mean? So I give the h3 an ID. The trouble i'm having now is that if I click the item more than once, I can't add other items. I can continue to add more to the initial item I add, though. I also noticed that if I add ONE to an item, I can then add them ALL. But as soon as I add more than one of the same item, I can't add more items of a different kind...
Thank you for your help, Steven
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThat's a different issue. But it happens because once you set "isDouble" to true, it never gets reset.
It would be better to set it explicitly either way each time:
Kristian Woods
23,414 PointsKristian Woods
23,414 PointsHey, Steven, apologies for the duplicate question. My code had actually changed slightly since the initial question, so, my question had slightly changed also.
However! my code works now. I can add new items and update the quantity of existing items. Thank you!.
I'm just still trying to wrap my head around why my code wasn't working before. I added the changed you suggested
Steven Parker
231,269 PointsSteven Parker
231,269 PointsAs I mentioned, the original code only changed the value to "true", but never set it back to "false". So once any item had been updated a second time, the code would never add a new one again.