Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial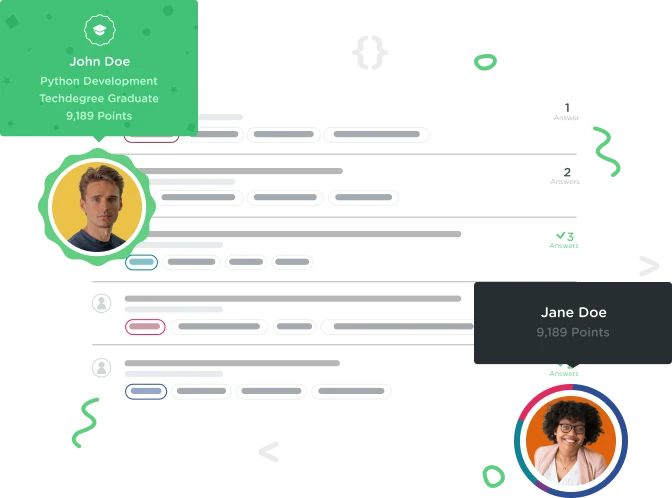

Joshua Rapoport
8,415 PointsWhy can't I use [1...10] ?
When initializing the allNumbers array, I found that I got an error when trying to use .filter() on it while having an array: let allNumbers = [1...10] The error disappeared as soon as I wrote the entire array out: let allNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] Does anyone know why that is?
1 Answer
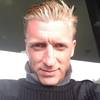
Rasmus Rønholt
Courses Plus Student 17,358 PointsThe 1...10 part creates what is called a sequence, and what you are doing is creating an array with one item only - that sequence. In this case it's just as easy to just type out the numbers, but if you do want to create an array based on values in a sequence, you can use the method map() on a sequence which, like filter(), is a built in higher order method that takes another method as an argument. In your case:
func returnIntUnchanged(i:Int) -> Int {
return i
}
let array = (1...10).map(returnIntUnchanged)
You don't even have to actually type out the function, but instead you can pass it directly as a block like so:
let array = (1...10).map({(i:Int) -> Int in return i})
Of course inside that function or block you can do anything you like with the sequence, and you don't have to return an int...
Ps. as it turns out, there are even simpler ways... but it's not a bad thing to understand the mechanisms above.
let array = (1...10).map{$0} //you can still "do stuff" to the values in the sequence here
let array = [Int](1...10)