Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial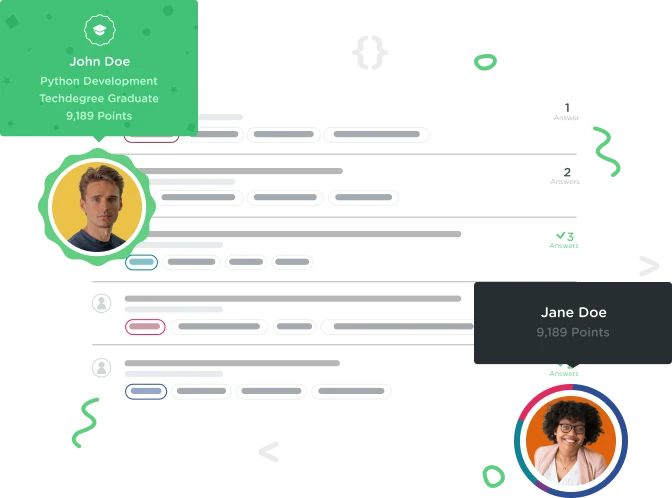

Julian Gu
12,490 PointsWhy can't I use a variable for count?
After testing this out in the shell, I have discovered that if I search for r"/w{4,}"
it works perfectly. However, if I instead search for r"/w{some_variable,}"
it returns nothing, even when some_variable has the value of 4.
Why does this happen and how do I fix this?
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, string):
return re.findall(r"/w{count,}", string)
6 Answers
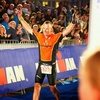
Steve Hunter
57,712 PointsI got this working by converting the count
integer to a string then concatenating that into the re.
def find_words(count, string):
count = str(count)
return re.findall(r"\w{"+count+",}", string)
With interpolation, I tried but was unable to figure out the solution:
def find_words(count, string):
count = str(count)
return re.findall(r"\w{{count},}", string)
# doesn't work
Steve.
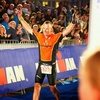
Steve Hunter
57,712 PointsHi Julian,
Did you try moving the comma?:
return re.findall(r"/w{count,}", string)
# try:
return re.findall(r"/w{count},", string)
Steve.
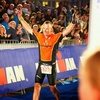
Steve Hunter
57,712 PointsDidn't work.
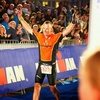
Steve Hunter
57,712 PointsCount isn't a string! Bear with me ...

Julian Gu
12,490 PointsThe only thing I can think of is that maybe I need to use the actual value instead of a variable which is technically the memory address (i think?), but I also feel like python does that automatically so I have no idea why this doesn't work.
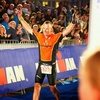
Steve Hunter
57,712 PointsI'm trying all sorts ... my latest fail:
def find_words(count, string):
count = str(count)
return re.findall(r"\w{{},}".format(count), string)
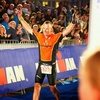
Steve Hunter
57,712 PointsThis might assist ...
Looks like concatenation is required, as in the answer below; not interpolation.

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsI ran into the same problem and it's pretty frustrating because this scenario isn't covered in any of the preceding videos.
I don't mind trying to figure out additional things, but Regex is arcane and confusing enough as it is, and I would have expected this to have been covered in the lead up videos.
All told, between trying different regex patterns and rewatching the videos to see what I might have missed, I spent about an hour on this problem. It's just an unnecessary waste of time.
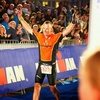
Steve Hunter
57,712 PointsI see your point but I don't think regex can be reasonably called arcane. It is well used and documented. I'd also say that something that makes you research outside of the course materials for an hour is teaching you way more than just watching the videos alone. I bet you're way more familar with regex than you were previosuly and much of that learning wasn't from the videos. That's a good thing in many ways.
Just my thoughts. I neither speak for Treehouse nor understand regex particularly well!
Steve.

Jimmy Smutek
Python Web Development Techdegree Student 6,629 PointsOkay, I'll concede that arcane wasn't the best word choice, and I'm definitely more familiar with regex at this point - but that is because of the material that's being taught in the videos and the exercises.
Sure, converting my integer to a string and concatenating it makes sense now, on the surface, but the point of the instruction at this stage is to learn how the sequences, characters and patterns work - at least that was what I was focused on.
I do understand your point. I spend a lot of time learning on my own, but unfortunately I didn't find that hour to be at all beneficial. It wasn't the good kind of research. It seems more like an oversight on the part of Treehouse, to be honest.
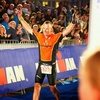
Steve Hunter
57,712 PointsIn fairness, I've not done these courses so can't really comment on their content. If you felt wholly unguided by the course videos then that's not ideal, obviously. Everyone learns differently, I guess - Treehouse has a narrow path to tread to meet everyone's needs.
Stick with it; you're clearly picking up lots so it isn't all fruitless.

Jimmy Smutek
Python Web Development Techdegree Student 6,629 Points:)
I'm getting older and can be cranky sometimes, but it's all good, and to clarify - despite some frustrations I can honestly say I do not regret having invested the time and money in this Tech Degree program. I'm learning tons, and Treehouse does a fantastic job, all told.
Thanks for the kind words.
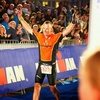
Steve Hunter
57,712 PointsI may try a Techdegree at some point. I have other certifications to chase first, though. Best of luck with it - I hope you get what you want from it.
Oh, I'm old and cranky all the time. And why not!
Steve.
Julian Gu
12,490 PointsJulian Gu
12,490 PointsI managed to got it to work by using
regex = r'\w{{{c},}}'.format(c=count)
But I got this from asking on Stack Exchange and still don't really know why this is necessary here.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsLooks like the concatenation is an easier way to maintain clarity. All those braces don't add readability. I did try with one set of nested braces; I have no idea what the requirement for a two-deep nest is, though.
At least it is possible if not recommended!
Good work!