Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial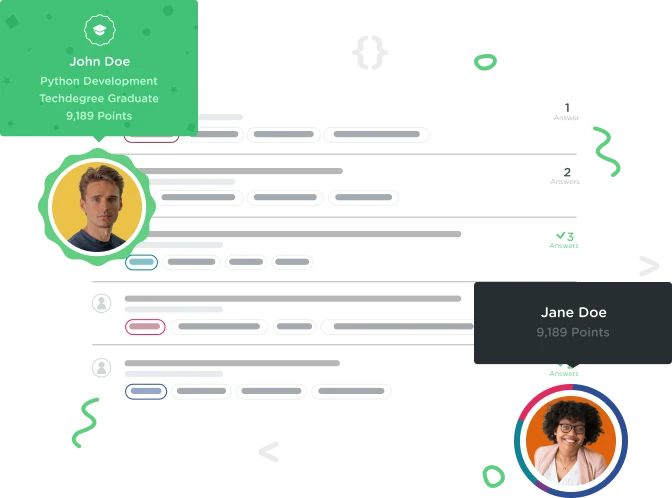

Kaytlynn Ransom
Python Web Development Techdegree Student 11,409 PointsWhy can't I use an if statement?
The exact question for the exercise is stated like this:
Correct the existing hasTile method to return true if the tile is in the tiles field, and false if it isn't. You can solve this a few ways, however, I'd like you to practice returning the result of the expression that uses the index of a char in a String.
I'm confused mostly by the last sentence. It tells me that it wants me to return true or false, but then it tells me that I need to return the expression using the index of a char in a String. How can I reconcile these two requirements to answer the problem correctly?
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean passedIn = tiles.indexOf(tile) != -1;
if (passedIn) {
return passedIn;
} else {
return false;
}
}
}
1 Answer
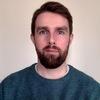
Richard Lambert
Courses Plus Student 7,180 PointsHello mate,
You can use an if-else statement:
public boolean hasTile(char tile) {
boolean isPassedIn = tiles.indexOf(tile) != -1;
if (isPassedIn) {
return true;
} else {
return false;
}
}
The following code also offers the same logic:
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
As you can see, creating an if-else statement introduces a lot of redundant code, but that doesn't make it incorrect. As to which implementation the Treehouse Java test engine will accept, I've tested the second and it passes; both make use of String
's .indexOf(char c)
method and return a boolean based on the presence or lack of a character in a given string, so should pass.
Hope this helps
Richard Lambert
Courses Plus Student 7,180 PointsRichard Lambert
Courses Plus Student 7,180 PointsUPDATE: Just tried this using an if-else statement and received "Bummer! While you could definitely solve this using an if statement, try returning the result of the expression." A case of the Treehouse Java test engine expecting a specific implementation.