Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial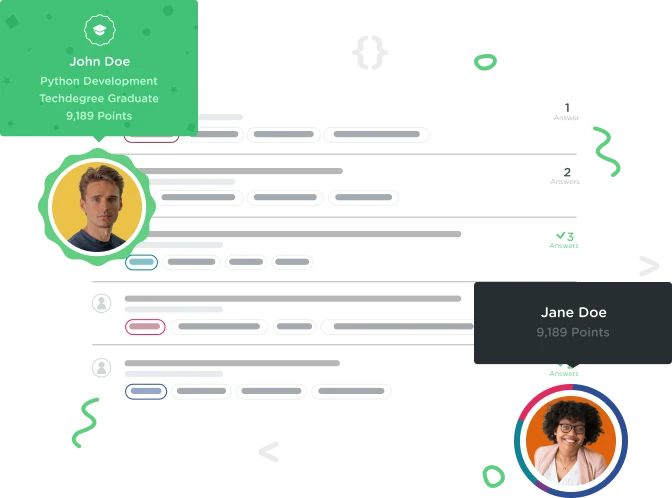
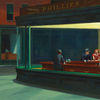
Anthony Grodowski
4,902 PointsWhy can't I use just self in __mul__ and __rmul__?
I've been discovering the usage of self and self.attribute and I thought I get it but...
class NumString:
def __init__(self, value):
self.value = str(value)
def __str__(self):
return self.value
def __int__(self):
return int(self.value)
def __float__(self):
return float(self.value)
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
return self + other
def __iadd__(self, other):
self.value = self + other
return self.value
def __mul__(self, other):
return self * other
def __rmul__(self, other):
return self * other
Why does it throw and error like this, when for example trying to multiply the instance times 3.2:
File "numstring.py", line 23, in __mul__
return self * other
[Previous line repeated 329 more times]
RecursionError: maximum recursion depth exceeded while calling a Python object
Code that gives me output as wanted is:
def __mul__(self, other):
return int(self.value) * other
def __rmul__(self, other):
return int(self.value) * other
but I don't understand why previous one gets into some sort of loop... TIA
1 Answer
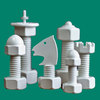
Steven Parker
231,141 PointsWhen you define "__mul__
", you are replacing the standard code for how to handle the " * " operator. So when you use that operator inside the definition, then the definition is depending on itself which causes the recursion.
But by using the " * " operator on an int value, you are invoking the multiply operation for int instead of the one for your own type (that you are redefining). So no recursion.
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsWhat do you mean by saying "definition"?
Steven Parker
231,141 PointsSteven Parker
231,141 PointsThe function (or method) "definition" is the code you create starting with the "def" keyword.
Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsThanks Steven! So putting int() causes redirection from
__mul__
to__int__
, which doesn't cause recursion. But I don't understand what means that "the definition is depending on itself which causes the recursion". How does it depend on itself?Steven Parker
231,141 PointsSteven Parker
231,141 PointsThe * operator calls the
__mul__
method to get the result. So if you use it inside the definition of__mul__
(on items of the same type), then it is calling (depending on) itself. But if you use a * to multiply items of a different type (like int), then it calls the__mul__
method that belongs to int instead.Anthony Grodowski
4,902 PointsAnthony Grodowski
4,902 PointsGot it. Thanks!
Steven Parker
231,141 PointsSteven Parker
231,141 PointsAnthony Grodowski — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!