Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial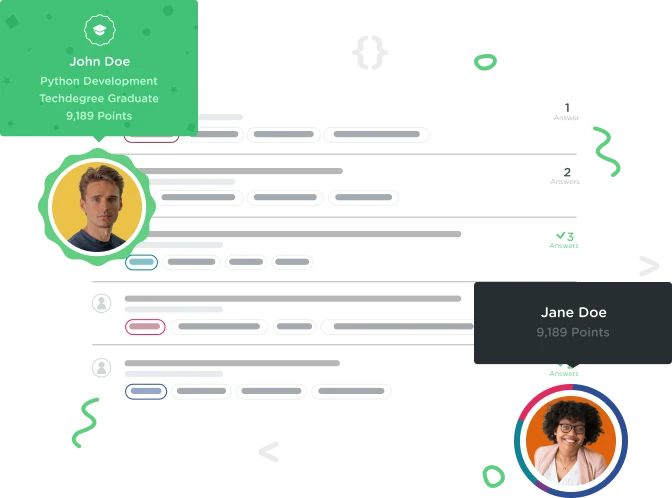

Darren Coburn
4,297 PointsWhy can't I use the instance of my class?
I created an instance of the tower class, but xcode won't let me use it. It doesn't even recognize it. I type "tower." and where it would usually give me options, it only gives me an error, "expected declaration"
class Enemy {
var life: Int = 2
let position: Point
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func decreaseHealth(factor: Int) {
life -= factor
}
}
class Tower {
let position: Point
var range: Int = 1
var strength: Int = 1
init(x: Int, y: Int) {
self.position = Point(x: x, y: y)
}
func fireAtEnemy(enemy: Enemy) {
if inRange(self.position, range: self.range, target: enemy.position) {
while enemy.life > 0 {
enemy.decreaseHealth(strength)
print("Enemy Hit!")
}
} else {
print("Sorry, the enemy is out of range!")
}
}
func inRange(position: Point, range: Int, target: Point) -> Bool {
let availablePositions = position.surroundingPoints(range)
for point in availablePositions {
if (point.x == target.x) && (point.y == target.y) {
return true
}
return false
}
}
let tower = Tower(x: 0, y: 0)
let enemy = Enemy(x: 1, y: 1)
// I want to use tower.fireAtEnemy(enemy) but Xcode won't let me use the instance in any //way
3 Answers
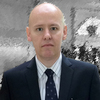
Nathan Tallack
22,160 PointsMissing a close brace "}" just before your return false on your inRange method. As a result you are not closing out your for point loop there in that method.
I hate it when that happens. Very very hard to see. Took me a good 5 mins to work it out, so don't be too hard on yourself. ;)

Darren Coburn
4,297 PointsYou are the best! You don't know how much I appreciate your help. But my next question is why didn't xcode pick that up? That was really weird. Thanks again!
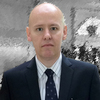
Nathan Tallack
22,160 PointsWell, it did kinda. That thing where it was saying let enemy was not working, that was because you were making that statement inside the scope of your Tower class.
I looked at your code in my browser for about 5 mins, could not see the error. Pasted it into a playground, saw xcode was saying consecutive declarations and immediately realised your let statements were inside the scope of the Class. That's when I counted the braces. ;)
So, when Xcode is giving you strange errors like that one, look at your scope. Often that is the answer. :)

Wilztan Andrew
Python Web Development Techdegree Student 4,979 Pointshi all i want ask what is the meaning of enemy:Enemy ? is it the enemy get the data from the Enemy class? and what is the meaning of enemy.position?
func fireAtEnemy(enemy:Enemy){ if inRange(self.position, range: self.range, target: enemy.position){
}
thx
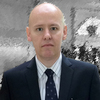
Nathan Tallack
22,160 PointsSo you are passing in a parameter to the method, in this case you are naming it enemy. So when you refer to enemy in the funciton block you are referring to that parameter you are passing in.
And Enemy is the type of object that you will be passing in as that parameter. It could be of type String or type Int, but in this case it is of type Enemy, which is the class you have defined. :)