Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial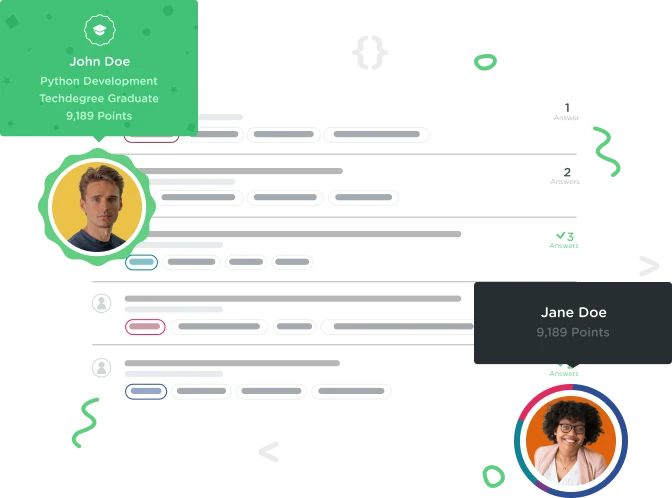
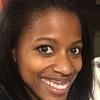
nicole lumpkin
Courses Plus Student 5,328 PointsWhy can't we access the value attribute after adding or multiplying in place?
Here is our class NumString:
class NumString:
def __init__(self, value):
self.value = str(value)
def __str__(self):
return self.value
def __int__(self):
return int(self.value)
def __float__(self):
return float(self.value)
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
def __radd__(self, other):
return self + other
def __iadd__(self, other):
self.value = self + other
return self.value
def __mul__(self, other):
if '.' in self.value or '.' in str(other):
return float(self.value) * other
return int(self.value) * other
def __rmul__(self, other):
return self * other
If I set an instance of NumString to a variable I am able to access the value attribute directly from age.
age = NumString(32)
age.value # this yields '32'
But if I increment age as defined via special methods add and iadd, the result is an AttributeError is raised.
age += 1
age # is now the integer 32
age.value #AttributeError: 'int' object has no attribute 'value'
Wouldn't we want to be able to access age's value attribute post in place addition? And if so is there a magic method that handles this case? This isn't mentioned in the videos but it's driving me crazy ;)
Thanks!
1 Answer
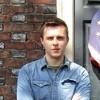
Kirill Babkin
19,940 PointsHey You answered your own question
age += 1
age # is now the integer 32
age.value #AttributeError: 'int' object has no attribute 'value'
age is now an int and not a reference to an obj NumString and int has no attribute value
as a result of this method you return an int or float:
def __add__(self, other):
if '.' in self.value:
return float(self) + other
return int(self) + other
^^^^ in the example above you return an int type or float type this makes age an int or float
try changing it in a way taht it would modify the obj and not return a number
def __add__(self, other):
if '.' in self.value:
self.value = float(self.value) + other
else
self.value = int(self.value) + other
return self
here ^^ you return a ref to obj.
it might not work thoug. Just demonstrating a concept ;-) Good luck!!
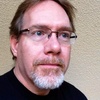
Chris Freeman
Treehouse Moderator 68,423 PointsChanged comment to answer.
nicole lumpkin
Courses Plus Student 5,328 Pointsnicole lumpkin
Courses Plus Student 5,328 PointsThank you Kirill! :)