Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial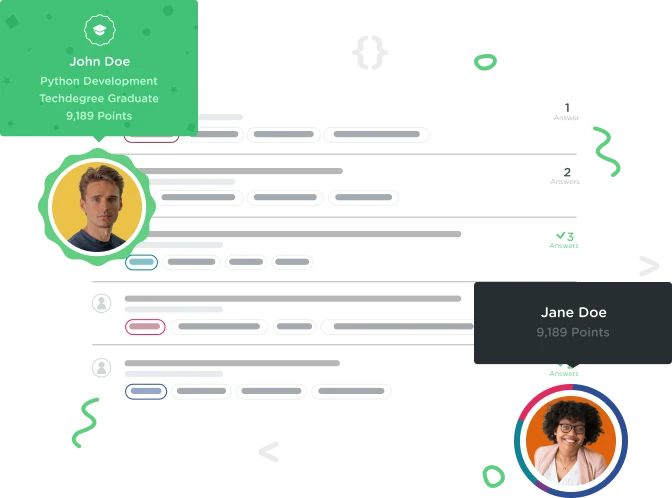
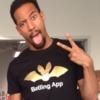
Mateo Sossah
6,598 PointsWhy can't we use -> todo_items.delete(name)
I tried the following and it is not working. Anybody knows why and how it could be fixed?
def remove_item(name)
todo_items.delete(name)
end
Thanks!
1 Answer
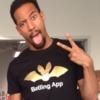
Mateo Sossah
6,598 PointsI found an answer using both the for loop and todo_items.delete(name). I still would love an explanation as why my initial solution didn't work and if this one is valid as it looks shorter than the one in the video:
def remove_item(name)
for todo_item in todo_items do
if todo_item.name == name
todo_items.delete(todo_item)
end
end
end
[MOD: added code blocks]
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsYour initial solution doesn't work because todo_items is an array containing objects of type todo_item; however what you are trying to delete from todo_items is not an object of type todo_item, but an attribute/member variable of such object.
The second solution works since what you are deleting from the list_items array is a todo_item object.
I would also suggest adding a couple of return statements to the method:
You could also use the each method instead:
Hope this helps.