Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial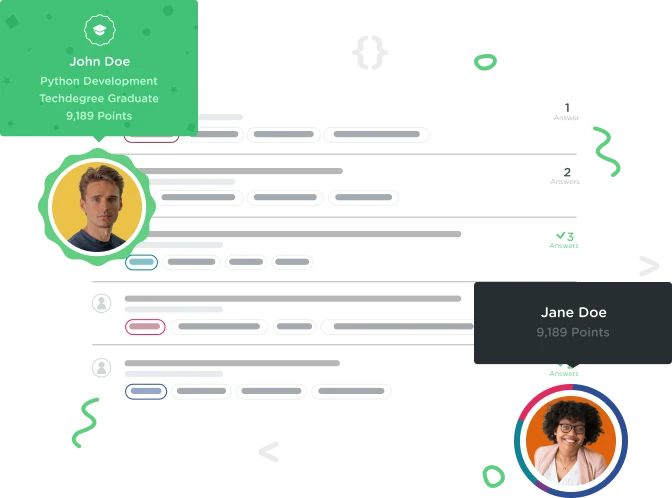
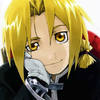
Gabriel D. Celery
13,810 PointsWhy diceRoll instead of diceRoll()?
There is something that confuses me. After we set up the function outside the constructor why are we referencing to it like this:
this.roll = diceRoll;
Instead of this?
this.roll = diceRoll();
If I try then it says this.roll "is not a function", so I know it has something to do with the fact that we are not calling the function, but referencing it, but it still doesn't tick.
3 Answers
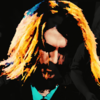
Justin Horner
Treehouse Guest TeacherHello Gabriel,
The reason it is referenced without parenthesis is to assign this.roll to the pointer of the diceRoll function. We want to be able to call roll as a function, so therefore it needs to point to a function.
When you add parenthesis, it is assigning the value returned from the diceRoll function to this.roll instead of assigning the function itself. This will cause code that is trying to calling the roll method to instead call a number as a method, which will give you errors.
I hope this helps.
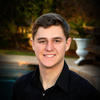
Colton Ehrman
Courses Plus Student 5,859 PointsExactly what Justin Horner said, this took me awhile to understand myself.
Basically you need to remember that in JavaScript function are objects, and can be used in tons of different ways.
One of the ways you can use a function is by using it as a "reference" to a variable. Which is what this.roll = diceRoll; does.
What this JavaScript statement does is... It will see the diceRoll "reference" it is a reference because you don't use the parenthesis, and then it will see the assignment operator.
It will then take whatever is on the left of the assignment operator and point that variable to the function reference on the right. Now, this.roll "points" to the function diceRoll and can be used as if it were diceRoll.
So really you aren't creating a new function with this assignment, but giving your previous function (diceRoll) a new "reference".
Now, let's look at the special parenthesis and see what happens when you use that.
So in, the statement this.roll = diceRoll(); JavaScript will see the function "call" since you have a function name (diceRoll) followed by the parenthesis, anytime you see this it is ALWAYS a function call.
It will then find the function diceRoll in memory and run whatever is inside the function block. If the function block has a "return" statement inside it, it will leave the block and return whatever the statement says. So for example if diceRoll has "return 10;" it will return the number 10.
Now for this return 10 example the statement this.roll = diceRoll(); will now look like this.roll = 10;
Because the function call will be replaced with whatever was return and this.roll will be assigned the number 10.
(Not really sure what happens if diceRoll doesn't have a return statement so you will need to look that up if you want to know, but I would have to guess it would be like assigning this.roll to nothing)
Anyways, hopefully this helped and didn't confuse you :) I'm glad to talk a little more about any part you don't fully understand!
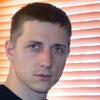
Lex Semenenko
9,906 PointsThank you for taking time. Great explanation, Colton Ehrman.
function sayName(name){
console.log(name);
}
var anotherReference = sayName; // not sayName();
// These two are the same
sayName("Lex"); // Lex
anotherReference("Lex"); // Lex