Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial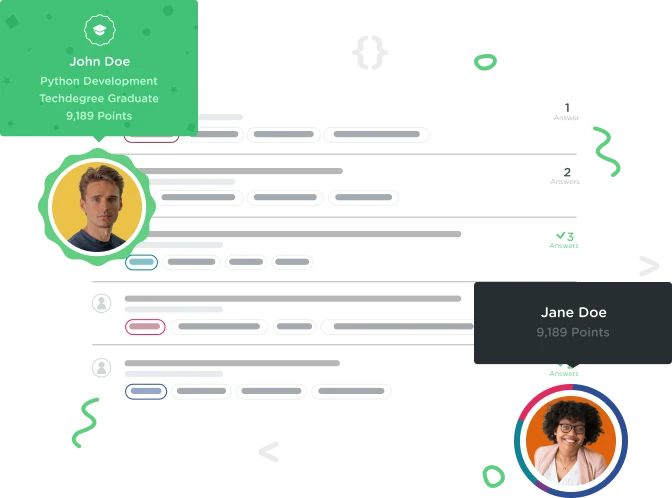
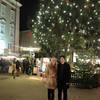
nicholas maddren
12,793 PointsWhy did Andrew use this.sides?
In the dice function Andrew uses this.sides like so:
function Dice(sides) {
this.sides = sides;
this.roll = function() {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
}
Why didn't he shorten his code by just including the sides parameter in the roll function? Like so:
function Dice(sides) {
this.roll = function() {
var randomNumber = Math.floor(Math.random() * sides) + 1;
return randomNumber;
}
}
I'm guessing there is a good reason behind this.
Thanks
3 Answers

Lars Reimann
11,816 PointsWhat you do here is called a closure. It means that even after the constructor function Dice has returned, the function this.roll still has access to the variables in the scope surrounding it when it was created, here sides. In this case I prefer your version. In general, however, you want to store any data directly in an instance and behavior in its prototype, which is shared across all instances of a constructor. Then you have to use Andrew's way:
function Dice(sides) {
this.sides = sides;
}
Dice.prototype.roll = function() {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsI am still learning but i think you cannot use sides on its own, you have to tell function that this.side to be in function is referring to sides passed in argument,or if you want to think of a car, all cars all gear but of you want to tell your car gear you will say javascript myCar.gear
not toyota.gear
, so you have to have reference to property or function i could be wrong.
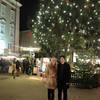
nicholas maddren
12,793 PointsIt works by just passing the argument 'sides' straight into the function. Thanks for the input though.

Mike Ngu
8,229 PointsIt does work both ways, but it's a better habit doing it Andrew's way. There's this weird thing in JS where if you wrap another function inside of that function the this keyword will point to the global scope.
Devjyoti Ghosh
2,422 PointsDevjyoti Ghosh
2,422 PointsJust to understand it properly, if this..sides is not set then during this example
In dice10 the sides is still set at 6 and not changed to 10?