Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial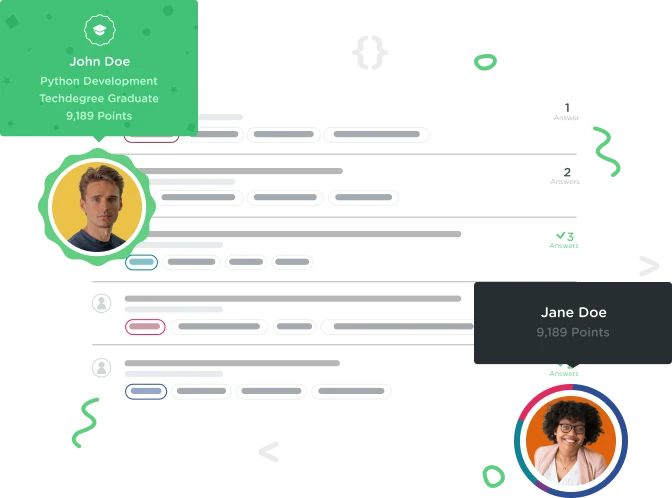

kj son
279 Pointswhy did he have to call err
so looking at the function, i am not quite sure why he had to call the ValueError to call up the one defined in the function.
From what I understand the function doesn't get called until it is initiated in the try statement, from there, the instructor inputs 20 and 0 respectively, 0 will trigger in the function and raise the ValueError which is "Oh no! That's not a valid value. Try again...". Then the next part is what confuses me, he calls the print function and prints the err variable, which then proceeds to grab the value error from the original function.
I don't quite understand what ValueError as err does, but why does it reference the valueerror in the function?
I hope my question makes sense.
6 Answers

Mark Maksi
1,451 PointsThe whole idea behind try, except and else is that they are meant to handle AUTOMATIC ERRORS OF Python. While Raise SomeError is meant to handle MANUAL ERRORS OF CHOICE and then run these manual errors in the except block.

Katie Ferencik
1,775 PointsThanks Mark - so if I'm understanding this correctly, then:
The automatic error happens because Python itself recognizes that an answer isn't able to be computed (i.e. answers as a string rather than a number that can then be coerced)
The raised error is when a user could enter something that can be coerced (such as a negative number), but logically it doesn't make sense (as in there can't be negative numbers of people), and we raise an error to prevent such a thing from happening.
Yes?

Mark Maksi
1,451 PointsKatie Ferencik exactly! We use "raise" to raise an error for things that don't work wit our program but Python has no problem with them. Automatic errors are those errors that are raised automatically by Python.
For example, Python automatically raises an error when you try to convert a string into an integer when you ask the users how many tickets they would buy. But nothing in Python prevents the users from entering a negative value because logically, negative values can be coerced into integers but in our special case, negative values don't make any sense because we need positive values for the numbers of tickets.

Katie Ferencik
1,775 PointsMark Maksi Awesome!! Thank you so much! It feels so good to "get" it now.

Jassim Alhatem
20,883 PointsGreat question,
def split_check(total, number_of_people):
if number_of_people <= 1:
raise ValueError("More than 1 person is required to split the check")
return math.ceil(total / number_of_people)
try:
total_due = float(input("What is the total?"))
number_of_people = int(input("How many people?"))
amount_due = split_check(total_due, number_of_people)
except ValueError as err:
print("That's not a valid value")
print("({})".format(err))
else:
print("each person owes {}".format(amount_due))
Let me break down what the program is doing in order:
- Takes the output of the first input and stores it in the variable total_due.
- Takes the output of the second input and stores it in the variable number_of_people.
- Calls the function and stores the return value in the variable amount_due.
Let me stop here for a minute. When the function gets called, it checks the number of people. If it equals or less than 1 it raises a ValueError("message"). It's called a raise exception(args). Therefore when you use that method, the args will be printed when you print the exception object. Example:
try:
raise ValueError("Raised an exception")
except ValueError as err:
print(err)
So what's happening is when the function is called and an error is thrown the args gets used (which is the message). Try inputting a letter instead of a number, it's not gonna throw the same error message because the function hasn't been called.
I hope this helps :)

Katie Ferencik
1,775 PointsI think I might have the same question as you (if I'm understanding yours correctly).
I'm wondering why we have two print statements that could be combined. Is it that the line
except ValueError as err:
prints something that could work for any part of the try (e.g. if the user inputs something other than an item that can be converted as a float, for example if they put in characters or letters)...
...and then the lines
if number_of_people <= 1:
raise ValueError("More than 1 person is required to split the check")
are specifically for the number_of_people.
It seems a bit more complicated than necessary.
So if the user gives an invalid answer to total_due, then it's going to give one error message. If the user gives an invalid answer to number_of_people, then it gives two error messages. Is there a reason for this?

Mark Maksi
1,451 PointsKatie Ferencik you're welcome! <3

Kylie Nonemaker
1,190 PointsI have the same question as Katie Ferencik above! Why would we want the error message created for when the user enters a string for when they enter a value that is <= 0?
Also, could someone explain to me how the following: except ValueError as err: print("Oh no! That's not a valid value. Try again...") print("({})".format(err))
Calls the value we set in: def split_check(total, number_of_people): if number_of_people <= 1: raise ValueError("More than 1 person is required to split the check") return math.ceil(total / number_of_people)
I've rewatched the video many times but I'm not getting it
Cody Stephenson
8,361 PointsCody Stephenson
8,361 PointsSo what is the purpose of handling entries that don't make sense as errors instead of just logically? So with a program that needs positive number input to make sense (but doesn't require it for the actual math) why is it better to handle it as
We want positive number input > if the user enters a negative we raise that as an exception > here is how we handle that exception and respond to the user with something useful
instead of
We want a positive number input > if the user enters a negative we respond with something useful.
It seems like the purpose of handling exceptions is that exceptions can actually break your program - why handle inputs that won't break anything that way just because they don't exactly make sense in the context of what the program does?