Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial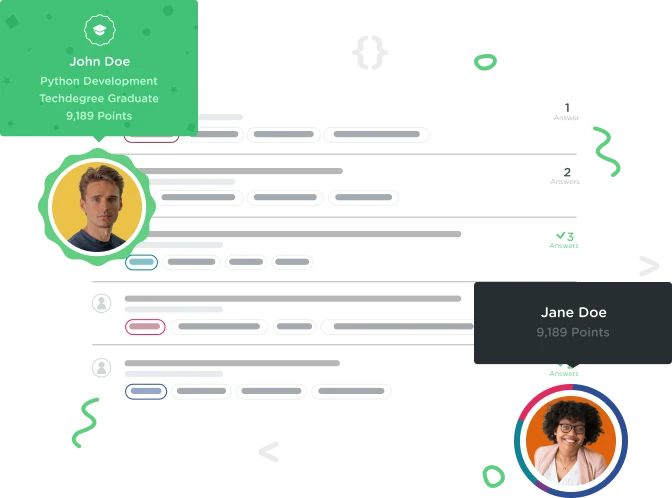

Paul M
16,370 PointsWhy did the code only put if (correctAnswer) instead of, if (correctAnswer = true) ?
I saw in the code this fucntion. My question is why did he leave out the = true statement after correctAnswer?
4 Answers

Nathan Crum
15,183 PointsThe if statement checks for true or false. If you are already storing true or false in a variable you only need to use the variable name inside the conditional statement.
var correctAnswer = false;
/*
* The variable contains false so the code inside the
* brackets will not run
*/
if ( correctAnswer ) {
...
}
/*
* This will set the value of the variable to true and run
* the code inside the brackets even though the first
* value was false
*/
if ( correctAnswer = true ) {
...
}
/*
* This will compare the value of our variable to true and
* return true if they match or false if they don't. could
* also use '==='
*/
if ( correctAnswer == true ) {
...
}
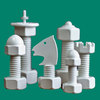
Steven Parker
230,274 PointsFirst off, for a comparison it would need to be == (double equals). One equal sign is an assignment.
But the whole purpose of a comparison is to determine a true or false condition. If a variable is actually storing a true or false, there's no need to compare it to anything, just test it directly.

Tim Renner
11,163 PointsThe part inside the parenthesis of an if statement is evaluated to a boolean. So if you supplied a statement like (correctAnswer == true), the value of correctAnswer would be inserted, i.e. (true == true) and that evaluates to true. If correctAnswer wasn't already boolean you could get unexpected results because the string 'true' is truthy and the value null is falsey, but the string 'false' is also truthy. So be sure to test your code for the intended behavior.
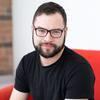
Garrett Levine
20,305 Pointsif you have a variable in an if statement, you're basically checking to see if that variable EXISTS. if it does exist, it will return true, if it doesn't exist, it will return false.