Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial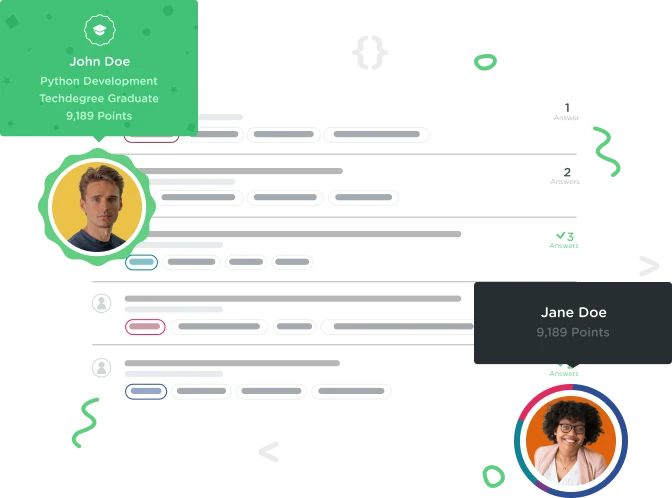

Unsubscribed User
1,785 PointsWhy do I always get: ValueError: list.remove(x): x not in list? HELP
Hey so I've been sitting on this for hours but I can't figure out why I always get the errormessage in the title, here is my code:
words = input("Type in some words, seperated by a comma > ")
word_list = list(words.lower())
vowels = ["a", "e", "i", "o", "u"]
if "," in word_list:
for items in word_list:
Commaindex = word_list.index(",")
word_list[Commaindex] == " "
for items in word_list:
word_list.remove(vowels)
joining = "".join(word_list)
print(joining)
Can you guys help me?
1 Answer
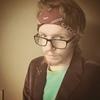
Jacob Murphy
Full Stack JavaScript Techdegree Graduate 32,014 PointsWhile I'm a bit unsure about what the whole commaindex thing is about I've been able to solve the error.
It lies within this code block:
for items in word_list:
word_list.remove(vowels)
joining = "".join(word_list)
print(joining)
Your problem is you're looping through each item in word_list and then trying to remove the vowels list, so your code is trying to remove a list that matches your vowels list, not each individual vowel.
The solution would be to loop through each vowel and remove them one-by-one like so
for vowel in vowels:
while True:
try:
word_list.remove(vowel)
except:
break
This way it goes through each vowel in the vowels list, tries to remove that vowel from word_list, and when all instances of that vowel is removed it breaks and continues the loop until it ends.
Hope this helped!
Unsubscribed User
1,785 PointsUnsubscribed User
1,785 PointsHey thank you so much, now I understand it! The commaindex thing is to get the comma (if there is more than one word) out of the list so I can print it without a comma. :)
One more question I have is: Is the continue in a while loop to go back to the beginning of the while loop?
Jacob Murphy
Full Stack JavaScript Techdegree Graduate 32,014 PointsJacob Murphy
Full Stack JavaScript Techdegree Graduate 32,014 PointsThe Continue statement, just tells a code block in a loop to continue with the next iteration of the loop, or next item its looping through. As opposed to the Break statement, which just stops the happenings of the parent loop. We use the break function here to exit the current
while True:
block so thatfor vowel in vowels:
can continue its loop. Continue really wouldn't work here because it would just let thewhile True
loop continue, and not exit, sofor vowel in vowels
would never move to the next iteration.