Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial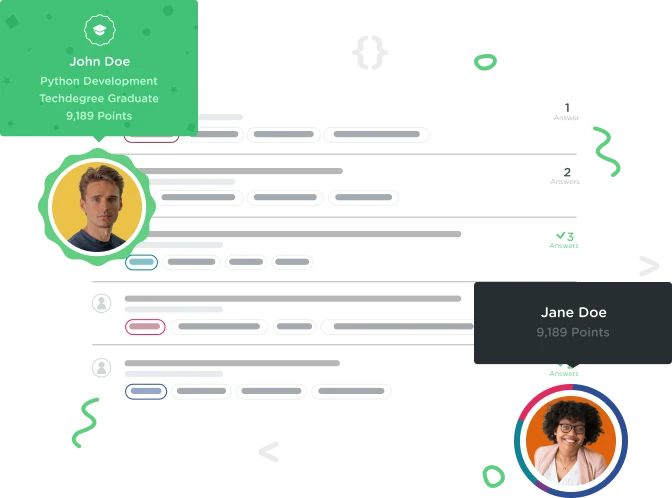
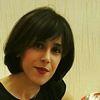
Sahar Nasiri
7,454 PointsWhy do I get an error?
def combo(it_1, it_2):
l = []
d = {}
for item_1 in it_1:
for item_2 in it_2:
if (item_1 not in d.keys()) and (item_2 not in d.values()):
d[item_1] = item_2
for key, value in d.items():
l.append((key, value))
return l
print(combo([1, 2, 3], 'abc'))
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(it_1, it_2):
l = []
d = {}
for item_1 in it_1:
for item_2 in it_2:
if (item_1 not in d.keys()) and (item_2 not in d.values()):
d[item_1] = item_2
for key, value in d.items():
l.append((key, value))
return l
print(combo([1, 2, 3], 'abc'))
1 Answer
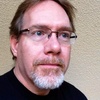
Chris Freeman
Treehouse Moderator 68,423 PointsThere are two issues with your code:
- if the order of the number is reversed, your code reorder them
>>> combo([3,2,1], 'abc')
[(1, 'c'), (2, 'b'), (3, 'a')]
- if there is a repeating character on the right, it gets dropped
>>> combo([1,2,3,4,5,6], 'abfdef')
[(1, 'a'), (2, 'b'), (3, 'f'), (4, 'd'), (5, 'e')]
Here are some alternate solutions to compare to:
def combo(itr1, itr2):
tup_list = list()
for index, num in enumerate(itr1):
a = num
b = itr2[index]
new_tup = a, b
tup_list.append(new_tup)
return tup_list
def combo(itr1, itr2):
tup_list = list()
for num, letter in zip(itr1, itr2):
a = num
b = letter
new_tup = a, b
tup_list.append(new_tup)
return tup_list
def combo(itr1, itr2):
# this can be shortened to:
tup_list = list()
for index, num in enumerate(itr1):
tup_list.append((num, itr2[index]))
return tup_list
def combo(itr1, itr2):
tup_list = list()
for num, letter in zip(itr1, itr2):
tup_list.append((num, letter))
return tup_list
# the last one can be shortened even further
# since 'zip' returns a the one entry from each list as a tuple
def combo(itr1, itr2):
tup_list = list(zip(itr1, itr2))
return tup_list
Sahar Nasiri
7,454 PointsSahar Nasiri
7,454 PointsThe answers were really helpful, thanks. :)